Calculate the Exponent (Solution)
00:00
As noted in the exercise instructions, we’re going to create helper variables for our computation and give them descriptive names of base
and exponent
.
00:10
They will both receive values from the user, so we need to call the input()
function on the right of each assignment operator to intercept the values.
00:20
The input()
function takes an optional prompt message as an argument which will be displayed to the user, so we can go ahead and add those prompts, "Enter a base: "
and "Enter an exponent: "
.
00:34 Notice that I’ve included a single space at the end of each message to give the users some breathing room. We can test this code by saving it and running the interactive shell on the left.
00:46
I’m going to provide the base and the exponent. This should have defined both variables in the current scope so I can access them by name. Let’s reveal the value of the base
variable.
00:59
As you can see, it’s stores a string, which we can double-check by calling the type()
function on the variable. To convert these values into numbers, we can call either the int()
function or the float()
one. In this case, it seems more appropriate to call float()
because we don’t know whether the user will provide a whole number or a fractional number, so it’s better to stay on the safe side. Let’s add the necessary conversion then. Now, since we’re in the numeric domain at this point, we can proceed to calculate the result, which is the base
raised to the power of the exponent
.
01:36 The last step is to print the output message, which we can craft using an f-string literal,
01:42
so f"{base} to the power of {exponent} equals {result}"
.
01:49 Notice that each variable that we refer to must be enclosed in curly brackets to replace it with the corresponding value. Otherwise, the string would just print the variable name literally. Okay.
02:01 Let’s give our program a spin. I’ll save it and reload it. I’ll provide the same values as before. Great. We’re done with this exercise, but if you feel like it, you can keep improving your solution—for example, by formatting the resulting number to limit the number of decimal digits.
02:21 There’s only one last section left in this course, which will let you practice a couple of math functions and number methods.
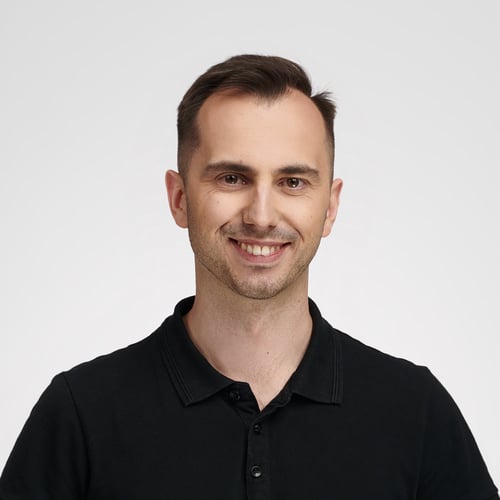
Bartosz Zaczyński RP Team on Jan. 19, 2024
@alvesmig Good catch. It’s a minor detail, which doesn’t matter as much, but I do like your solution! By the way, you have a typo in your variable name: exponant
🡒 exponent
😉
Become a Member to join the conversation.
alvesmig on Jan. 19, 2024
Hello,
nice exercises to complete course.
Your output doesn’t match exactly the output in the exercise description. In the exercise description the output was:
Your output is:
Here is my solution to have the same output as in the exercise description:
Best regards
Miguel