Catching a Built-in Exception
00:00
In the previous lesson, you ran into a new exception, the FileNotFoundError
, and this is a built-in exception and you already know what to do.
00:08
You’re going to go and handle it using a try
… except
block. But first let’s take a look at this FileNotFoundError
in the context of the other built-in exceptions that exist.
00:17
And this is a long list. I took it from the documentation, and I had to cut it up because there’s so many built-in errors. You can see also how they all inherit from the BaseException
, and then there’s this Exception
class that you used towards the beginning of this course.
00:32
You can pause this video for a second and see if you can find the FileNotFoundError
. I’ll give you a second for that. And now let’s head back over to the code and handle the FileNotFoundError
, make sure that the program doesn’t quit if the logging file doesn’t exist.
00:51
So back over here, let’s add another message. Copy this just to say that we’re reading the logging file. And then you’re attempting to open a file that doesn’t exist, so you want to wrap this again into a try
… except
block. I’m going to say “Try to open this file,” and then, “Print out the log file,” and “except
if you have a FileNotFoundError
,”
01:17 and you can again assign it to a variable,
01:22
then you want to print out "Oops"
01:29 and maybe add an emoji to it,
01:35 and print out also the error message.
01:42
Okay, so this is the common try
… except
block that you can use often and that you will often see used in Python code, where you expect a certain exception to occur and then you do something if it occurs indeed.
01:57
So now I run this code. You will see that the important macOS work gets done. There’s no problem, the work happened, everything was great. No exception occurred, which is why the code inside of the else
block started running.
02:16
It tells you that it’s going to start reading the logging file. But now, in here, you have another try
… except
block where it attempts to open this file, and it doesn’t find the file because it doesn’t exist.
02:27
But instead of ending the script, you’re catching this FileNotFoundError
that comes up and printing out a message plus also the error message that happened before. And your script continues, which means that also this "happens after"
still prints after that.
02:43
And this is how you can catch any sort of exception in Python, be it one that you defined yourself, like you did here with the WrongOsError
, or just one of those built-in ones like the FileNotFoundError
. And again, there’s a lot of built-in exceptions, and it generally makes sense to just use one of those built-in exceptions instead of making and subclassing your own. Just make sure that you know which ones can get raised and in which condition you want to catch what exception. This is how you can catch built-in exceptions in Python.
03:17
In the next lesson, you will look at yet another keyword that you can use with the try
… except
block, which is called finally
.
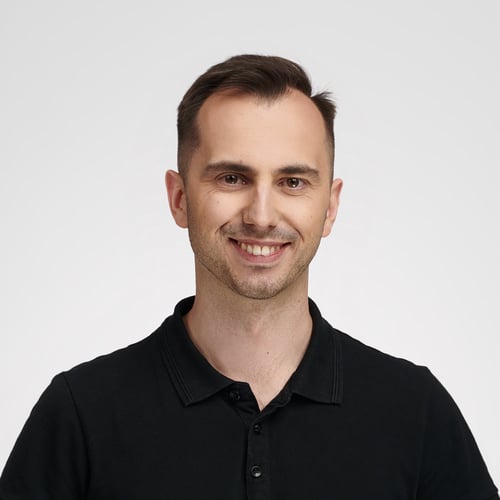
Bartosz Zaczyński RP Team on Jan. 3, 2022
@torrepreciado As a rule of thumb, the scope of a try..except
clause should be as short as possible, so nesting makes sense. If the scope encompasses more code, then you might end up catching exceptions raised in places you weren’t expecting.
Wrapping the nested code in a function sounds even better to me, but the question remains where to handle that exception. Should the function be responsible for that or would you rather leave it to the higher-level code?
Become a Member to join the conversation.
torrepreciado on Dec. 31, 2021
Would the nested use of the
try..except
be discouraged here? Would we be better off by wrapping the secondtry..except
into another function?