Composition
00:00 Let’s change gears and talk about composition now. Composition models a has a relationship between classes.
00:10 You could also think of this as a part of relationship. Just like before, let’s think of some examples of composition in the real world. I came up with a car and an engine.
00:25 A car is composed of an engine. In other words, an engine is a part of a car.
00:33
This is how this might look in code. Here, we have a Car
class. The car has some pretty standard attributes, such as a brand, a model, and a year.
00:46
We can probably guess what types those attributes should be. Brand and model are strings, and year is an integer. But what type should the engine be? Python has no built-in Engine
type, so here’s an opportunity for composition.
01:04
We create a separate class called Engine
, which has attributes like the number of cylinders, some efficiency rating, and a weight. These can all be integers.
01:16
Now, we can say that our car’s .engine
attribute should be of type Engine
, meaning that the .engine
attribute must be set to a valid Engine
object. Because the Car
will have a valid Engine
object at the time it’s created, we can add some code in the car’s .turn_on()
method that literally calls the .ignite()
method in the car’s Engine
.
01:42
We can even access the Engine
from a more global scope. For example, we can create a Car
object called my_car
, supplying a valid Engine
object for its .engine
attribute, and then get to the .weight
of that Engine
from the global scope by saying my_car.engine.weight
. Once again, I’ve prepared a few questions to test your understanding, so go ahead and pause the video and try your best at answering them.
02:17
The first question asks, “What is the type of the .engine
attribute?” That is simply Engine
. Remember, a class’s attributes are simply variables that objects instantiated from the class keep track of.
02:33
We can name variables virtually anything, and that’s completely independent of the variable’s type. I could have called the .engine
attribute .motor
, or .xyz
, or anything you could normally name a variable.
02:49
The only way we can tell the type of the .engine
attribute in this diagram is because I drew a big arrow that says part of from the Engine
class to the bolded word engine. In a later video, you’ll learn about a more formal approach to diagramming these classes.
03:09
Next, I asked, “Does the .accelerate()
method have access to the .efficiency
attribute?” Here, the answer is yes. Remember, the methods you can call on an object have access to the attributes of that object.
03:27
That means that the .accelerate()
method can access the car’s .engine
attribute, which itself has an .efficiency
attribute.
03:36
The last question says, “Can the .ignite()
method in the Engine
class access the .brand
attribute?” It cannot, and that’s because this is composition and not inheritance.
03:49
You could say the Engine
class is blind to where it’s being used, and so it doesn’t have access to any of the inner workings of the Car
class.
03:59
It’s its own independent entity. We could also use the Engine
class in other classes if we wanted. It’s not strictly tied to the Car
.
04:11 Before I finish off this video, I want to introduce a little bit of terminology regarding composition. In Python, one class can be a component of another composite class.
04:24
This means that, in the last example, Car
was the composite class that was made up of an Engine
component. In the next video, you’ll see some examples of how inheritance is used in various parts of the Python language.
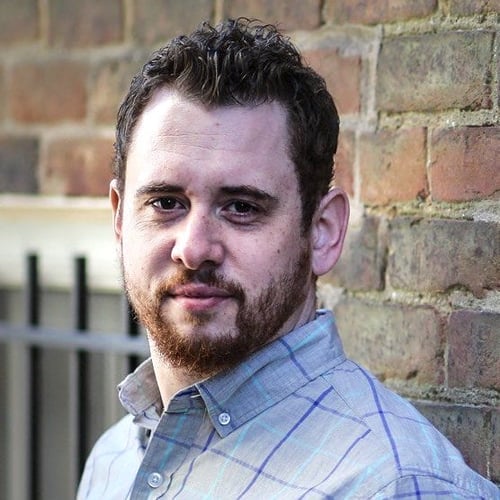
Ricky White RP Team on May 1, 2020
While we don’t have a quiz for this topic yet, we do have them for others if you wanted to test yourself. You can find them all here: realpython.com/quizzes/
muondude on Jan. 12, 2022
Why are you saying that the Car
and Engine
relationship is one of composition? I thought composition implied that the child does not exist without the parent (house - roof)? Wouldn’t a Car
-Engine
relationship be aggregation - the engine can exist independent of the Car
?
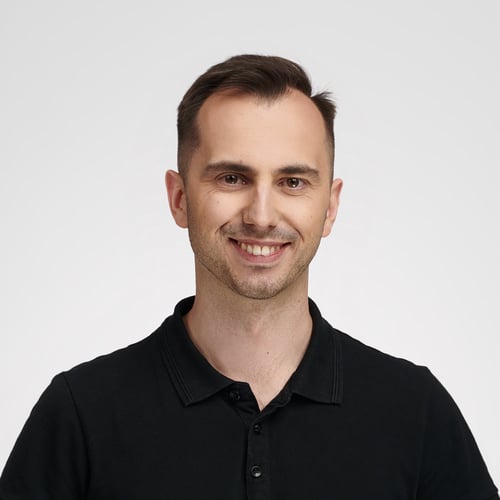
Bartosz Zaczyński RP Team on Jan. 13, 2022
@muondude You’re technically right about the distinction between aggregation and composition. However, in everyday speech, “composition” is often used informally in both meanings.
Mariusz Kula on Feb. 3, 2023
I think to avoid confusion in the following question the type should be capitalized as in:
Q. What is the type of the engine attribute?
A. Engine
That is because the type is defined by the class which is ‘Engine’ and not ‘engine’. ‘engine’ is an attribute whose type is ‘Engine’.
Become a Member to join the conversation.
fjavanderspek on May 1, 2020
Thanks for the great videos! I like that you ask us questions so we can test our understanding. This really fortifies the understanding we have, exposes gaps we may still have, and helps commit the information to memory! Keep it going :D