Daemon Threads
In this lesson, you’ll learn about daemon threads. The main difference between a regular thread
and a daemon thread is that the main thread will not wait for daemon threads to complete before exiting. If you download the sample code, you can get your own copy of 04-daemon.py
:
To learn more, you can also check out the documentation.
00:00
In the previous lesson, you created a thread and you saw how the main thread didn’t wait for the new thread to finish before it printed that final line main ended
.
00:11
Sometimes we do want the main thread to wait for all of our threads to finish before we hit that final line in our program, and one way we can do that is by using daemon
threads.
00:23
daemon
is a word referring to a background process or something that kind of hums along silently in the background while you’re doing something else.
00:33
An example of a daemon
process could be something like maybe a Django web server that you’re running in the background, that when you’re down in your terminal, you don’t see it running, but you know that you can hit localhost:8080
or whatever and access that. So, it’s a process that’s running, but it’s not necessarily up front and in your face all the time.
00:54
It’s a daemon
process, it’s in the background. For this example, I’m actually just going to use the exact same code from our previous lesson.
01:03
I’m going to copy that and just bring it into a new file. I’m calling mine 04-daemon.py
.
01:11
And we’re just going to make a slight difference, but before we do that, let’s execute our program again. This time I’ll do 04-daemon
and just verify—yep, we’re seeing main ended
from our main thread while t
is sleeping, up here. And then we should see myfunc ended
here in a moment—there it is, and we’re back to our prompt.
01:35
This time we’re going to add one more argument to this threading
object and it’s called daemon
. And by default it’s False
, but we’re going to make it True
and see how this affects our code.
01:53
Okay, so right away we see a difference. There was no sleep()
. The execution was really fast. So let’s break it down, let’s see. This is our __main__
entry point, so we see main started
.
02:05
Then we created t
and this is now a daemon
thread. So we started t
,
02:14
t
went up and it printed myfunc started with realpython
and then it went to sleep. And while t
was asleep, then the main thread was able to continue on and it printed main ended
, but there was no sleep()
there, and I’ll execute it again. There was no sleep()
.
02:31
It didn’t get a chance to finish. By adding daemon=True
, it doesn’t let the thread necessarily finish before the main thread ends.
02:43
What daemon=True
provides is the ability to end our program when the main thread ends, but that could be dangerous because maybe our threads—and we only have one extra thread, but we might have many threads—maybe these threads aren’t done doing what they need to do, and it can have a very abrupt ending, like we’re seeing here.
03:07
We don’t get to sleep for 10 seconds. So, sometimes you want to do this, and sometimes you don’t. But this is an option—by adding daemon=True
, you can do this. In later videos, we’re going to learn how we can actually wait for threads to complete before we finish our main program.
Aniruddha on Nov. 8, 2022
So why do we have deamon thread if it does not let finish other thread?
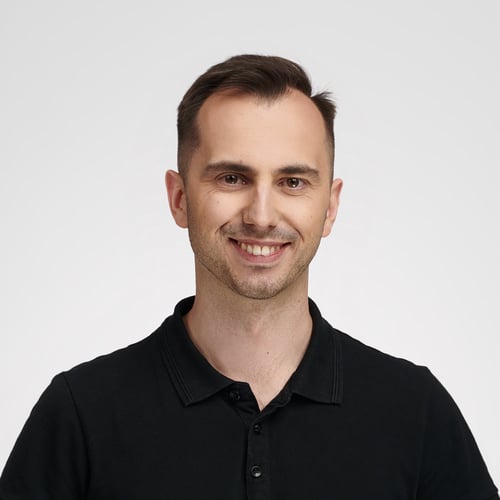
Bartosz Zaczyński RP Team on Nov. 8, 2022
@Aniruddha Regular threads keep the Python interpreter running as long as there’s at least one non-daemon thread alive. On the other hand, Python doesn’t wait until your daemon threads finish. The interpreter will stop them abruptly when the last non-daemon thread—usually the main thread—finishes, even without letting the try..finally
clause run, which would usually execute unconditionally. Therefore, you should never acquire any resources in daemon threads to avoid memory leaks and other problems such as deadlocks. They’re best used as lightweight background tasks, for example, to monitor something or send a heartbeat to a monitoring software.
Become a Member to join the conversation.
Alvaro Formoso on April 2, 2020
Hello! One quick question from my side: In the previous video, without using Daemon, for me (With Spyder Interpreter and Python 3.7.1) it was doing already the behaviour that you got with daemon=True. I mean, I could write in the console more things just after Main Ended sentence is written on the console.
Is that an update from the library?
Thanks! And Good job, the webpage is very cool! :)