Documenting With Docstrings (Part 2)
Docstrings have different layouts depending on what they’re being used for. The content, however, generally stays the same.
To learn more about docstrings formats, check out the following resources:
00:00 Welcome to Part 5 of the Real Python tutorial for Documenting Your Python Code. Last time, we left off after defining the major categories of docstrings.
00:09 Let’s explore them now. Let’s start by looking at a class docstring example. As you can see, it’s reasonably straightforward at this point. You just place the string literal directly below the class or the class method, depending on what you’re writing the docstring for.
00:28 And that’s the basic docstring. Class docstrings are created for the class itself, as well as any of its class methods. The docstrings are placed immediately following the class or class method, indented by one level, which was shown on the previous example.
00:45 Class docstrings should contain the following information: a brief summary of its purpose and behavior, any public methods along with a brief description, any class properties and attributes, and anything related to the interface for subclasses if the class is intended to be subclassed.
01:06
The class constructor parameters should be documented within the .__init__()
class method docstring. Individual methods should be documented using their own individual docstrings. Class method docstrings should contain the following: a brief description of what the method is and what it’s used for; any arguments—both required and optional—that are passed, including keyword arguments; label any arguments that are considered optional or have a default value; any side effects that occur when executing the method; any exceptions that are raised; and any restrictions on when the method can be called.
01:48
Now we can look at an example. This data class represents an animal. The class contains a few class properties, instance properties, a .__init__()
property, and a single instance method.
02:01 If we go have a look through, we can see each one of them in turn.
02:07
You can see the triple double quote ("""
) right there to begin the docstring, and clearly it’s a multi-lined docstring. You can see here that it explains each attribute—what it is and a very, very brief description per attribute.
02:26 The method that it contains also has its own docstring.
02:32
And then within the .__init__()
method, you have its own docstring, as explained earlier. If we look down at the very bottom here, then you can see
02:45
an error that gets raised if there is no sound for the animal, and you can say here that that is called out specifically within the docstring, again as mentioned earlier it should. Let’s move on to package and module docstrings. Package docstrings should be placed at the top of the package’s __init__.py
file.
03:08 This docstring should list the modules and sub-packages that are exported by the package. Module docstrings are very similar to class docstrings, except instead of classes and class methods being documented, it’s now the module and any functions found within. Module docstrings are placed at the top of the file even before any imports.
03:28 Module docstrings should include the following: a description of the module and its purpose, and a list of any classes, exceptions, functions, and any other objects exported by the module.
03:41 Otherwise, the docstring for a module function should include the same items as a class method docstring that were indicated earlier. These are, of course, a brief description of what the function is and what it’s used for; any arguments—both required and optional—that are passed, including keyword arguments; label any arguments that are considered optional or have a default value; any side effects that occur when executing the function; any exceptions that are raised; and any restrictions on when the function can be called. Scripts are considered to be single file executables run from the console. Docstrings for scripts are placed at the top of the file and should be documented well enough for users to be able to have a sufficient understanding of how to use the script.
04:25
It should be usable for its usage message when the user incorrectly passes in a parameter or uses the -h
option. Finally, any custom or third-party imports should be listed within the docstrings to allow users to know which extra packages may be required in order to run the script.
04:44
Now, as a quick side note, if you use argparse
, then you can omit parameter-specific documentation—assuming, of course, it has been correctly documented within the help
parameter of the argparse.parser.add_argument()
function.
05:00
It is recommended to use the .__doc__
for the description
parameter within argparse.ArgumentParser
’s constructor. For more details on how to use argparse
and other common command line parsers, you can visit the Real Python tutorial on Command-Line Parsing Libraries.
05:16 We can now look at an example. Here is an example of a script which extracts column headers of a spreadsheet.
05:24
If you’d like to peruse this at your own pace, feel free to either pause the video or check out the written version of this tutorial on the Real Python website. If we briefly go through this together, however, you can see that it gives you a brief description of what it’s meant to do and any assumptions that are made in order to make the script work. Here, you can see that it tells you it accepts different types of files, and it also tells you that it requires pandas
to be installed within the Python environment you’re running. That, again, covers the rule that it should tell you what other third-party programs or packages are required to run the script correctly.
06:01
And then you can see the imports coming in after the docstring, and then you can see the docstring of the method here, get_spreadsheet_cols()
.
06:11 Just like any other docstring, it comes directly below, indented by one level.
06:19 And there you have it! The script docstring.
06:23 The final aspect of docstrings is formatting. Throughout this tutorial, all the examples have had specific formatting with common elements: arguments, returns, and attributes.
06:39 There are specific docstring formats that can be used to help docstring parses and users have a familiar and known format. The formatting used within these examples is the NumPy/SciPy style docstrings, which we’ll get to in a moment. Some of the more common formats are Google docstrings, which is Google’s recommended form of documentation.
07:01 If we have a look at an example, now you can see how the arguments and the returns are set out differently to all the previous ones that we’ve seen in this tutorial.
07:12 We also have reStructured Text, which is not considered to be beginner-friendly, but it is quite useful. It’s also the official Python documentation standard.
07:25 You can see the layout here. It includes everything that the Google docstrings format has, it’s just laid out quite differently, as you can see in the example here.
07:37 We also have NumPy/SciPy docstrings, which, as mentioned, is what we’ve been using so far in this tutorial. This is a combination of Google docstrings and reStructured Text, so we should be quite familiar with the docstrings example here.
07:55
And finally, we have Epytext, which is a Python adaptation of Epydoc. This is a very good option for Java developers. If we have a look here, we can say why, with the at (@
) in front of each type and parameter.
08:12 It’s a very Java-style structure. Now, which format you choose is entirely in your own hands. However, once you choose one, it should be used throughout your entire document or project.
08:26 There are some really good resources available for all of these examples that I’ve just gone through on the Real Python written tutorial for documenting your Python code, so please feel free to go check that out.
08:41 Join me in the next video, where we will cover the final aspect of this tutorial: Documenting Your Python Projects.
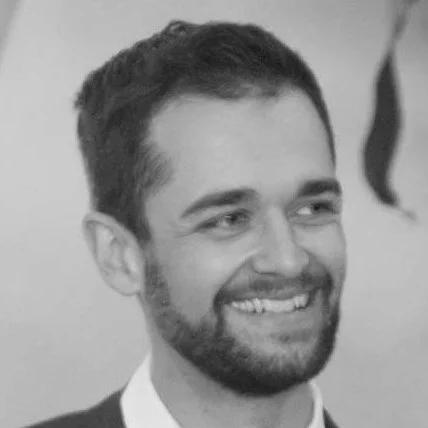
Andrew Stephen RP Team on March 12, 2020
Hi @ron53white it is actually pronounced “dunder” and it refers to any variable that has leading and trailing double underscores, e.g. name would be pronounced “dunder name”, main would be “dunder main” etc. I mention this @2:20 in the previous video (Documenting with Docstrings (Part 1)).
Become a Member to join the conversation.
ron53white on March 12, 2020
I’m trying to figure out the repeated references to “dundun”. Was this defined elsewhere and I missed it? It’s been used in several sections. Or maybe I’m just not hearing it correctly given differences in diction.