The == Operator
00:00
In this lesson, I’ll cover how to compare equality with the double equals (==
) and not equals (!=
) operators. Luckily, this form of comparison is a lot easier to understand and has far fewer kind of quirks or idiosyncrasies than the is
and is not
operator.
00:18 And the reason for this is that it kind of conforms to our expectations about what equality means when we think about equality out in the world of non-computers. You know?
00:27 Normally, we’re not just comparing some ID numbers—we’re comparing some kind of inherent quality of the objects being compared that makes them equal, in a sense that’s interesting to us.
00:39 And I’m starting to get back into kind of the realm of philosophy here, talking about inherent qualities of objects and so forth, but picture something like you’re going to a hardware store and you need a length of wood or something to build a fence or something like that, right? Now, you might care about the particular piece of wood that you want, but it’s unlikely that you really care whether you have piece of wood X or piece of wood Y. What you really care about are a small set of predefined variables about the wood, right? You care about the kind of wood, whether it’s oak, or elm, or a cedar maybe. And you care about the length, you care about the width, the height, so on.
01:20
So you care about some set of qualities of the object, right? And so that’s the definition of comparison that the equality comparator makes use of, and the not equals (!=
) as well.
01:33
And this definition of comparison, unlike is
and is not
, varies between different kinds of objects being compared, right? So a numeric comparison is very different from a string comparison, which is also different from a comparison between two kinds of customers of an online store or something like that, right?
01:53
And how that’s implemented under the hood—and what I’ll cover in much greater detail in another lesson—is the .__eq__()
, or the equals, method of the class definition of an object.
02:07
So, let’s get over right into the terminal and it’s there that I’ll cover when to actually use this equals operator (==
), though I think you probably have some good ideas of that already.
02:17
So, let’s take a quick look again at some string comparisons. If I have a = "This is a string"
and b = "This is a string"
,
02:28
then I talked about in the last video about how a is not b
, right? a
and b
are not the same object in memory because their IDs are different.
02:38
ID of a
and ID of b
are in fact different numbers. But a == b
is True
, and this is simply because the equality comparison, the class method of string which compares equality, just goes through character by character and compares whether each individual character is the same. So if I said something like a = "Thisisastring"
with no space between "This"
and "is"
, and then I just had the b
, which is "This is a string"
, then a == b
would be False
and a != b
would be True
.
03:15
And of course a is b
is still False
because they’re different objects, right? So, a way that this might be more obvious would be to show with a list copy example.
03:28
So if I have a
as the list [1, 2, 3]
, b = a.copy()
—and this is a convenience function that lists offer, just copy the contents of the list. So, if you look at a
and b
, they now have exactly the same values in them. And lists define this ==
sense of equality by just comparing the values of the list, so a == b
is True
, and correspondingly, a != b
is False
.
03:55
But a
is not b
, right? So a is not b
is True
. You can see all four different kinds of comparison. And so that brings up a point about double equals (==
) and not equals (!=
), is that the two should be inverse of each other, and if they’re not inverse of each other, that’s a bug in the equality method of the programmer who’s defined that class. Because, really, you need those things to be True
.
04:19
And then ==
should pretty much always be used when you are doing a literal comparison. So 44
, if you want to compare 44
and 11 * 4
, you should always use the ==
because that’s kind of the numeric comparison and will almost always be something that you should use the ==
for. Because saying, you know, 44 is 11 * 4
doesn’t even make sense, really.
04:44
It’s kind of like, what—these are not even stored objects, or not even objects that you’ve assigned a storage to, so that’s why you get this SyntaxWarning: "is" with a literal
.
04:54
And, of course, I did mean ==
in the sense that that’s what I should have used. So, that is the double equals (==
) and not equals (!=
) operators, and the important thing to keep in mind with this form of comparison is that it compares by equality, and what that means really is the content of the object, rather than the actual identity of the object.
05:17 So, to think again about maybe another real-world example that might be helpful is imagine that you have two different boxes and they contain something in them, right? If you have a box full of candy and then a box full of toys, those are clearly not equal in that they don’t have the same contents, right?
05:37
And it’s also true that one is not the other because they are not the same exact object. But if both boxes have candy, you might say in some meaningful sense, in the sense that the ==
operator works, the two are equal to one another because they’re the same size, the same shape, they contain the same stuff. Right?
05:55
So, that’s the idea behind the equality operator. And in practice, this equality comparison operator is the one that you should be using far more frequently, unless you’re comparing to None
, like I mentioned in the is
video.
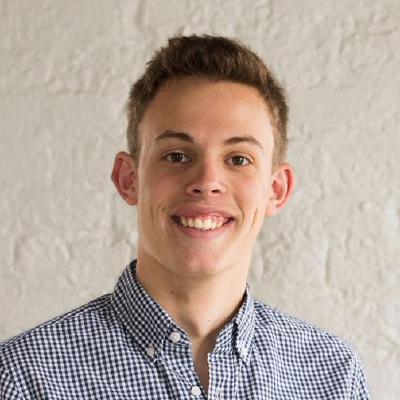
Liam Pulsifer RP Team on Aug. 11, 2020
Hmmm, I see where the disturbance comes from @Zarata, but I think a return value of True
is still the best possible option here. The is
operator answers a very simple question in Python – do the two operands have the same id
value? In this case, they do because of the interning system that Python puts in place, so the expression returns True
. The reason that it’s a warning is because while it’s a logically consistent result, it’s not clear why anyone would want to use it. An error would imply that the is
comparator can’t be applied to these two operands for some reason, when in this case that’s not really true. Of course, reasonable people can disagree on this, so if you were to implement your own programming language, it would be perfectly reasonable to make this kind of thing a SyntaxError. I think though that this fits under Python’s relatively permissive approach to operations in general: Python tries not to explicitly prohibit you from doing things unless it would cause a direct error or impossible result.
Zarata on Aug. 11, 2020
I guess I’m not Dutch :)
Become a Member to join the conversation.
Zarata on Aug. 11, 2020
It’s slightly “disturbing” that despite the SyntaxWarning for
44 is 11 * 4
the REPL still returnsTrue
. This return muddies the point you are making. What is the meaning of theTrue
return in this case? (For that matter, why is this flagged as a Warning and not an out-and-out Error?)