Python Basics Exercises: Object-Oriented Programming (Summary)
In this course, you practiced what you learned in Python Basics: Object-Oriented Programming. You’re ready to use object-oriented programming (OOP) to write more readable and maintainable code in Python! You can now confidently create blueprints for objects that contain both data and behaviors.
In this video course, you’ve practiced how to:
- Create a
class
- Use classes to create new objects
- Instantiate classes with attributes and methods
For more information on the concepts covered in this course, check out the following tutorials:
- Object-Oriented Programming (OOP) in Python 3
- Intro to Object-Oriented Programming (OOP) in Python
- Getters and Setters: Manage Attributes in Python
- Operator and Function Overloading in Custom Python Classes
Or you can explore the following video courses:
- Intro to Object-Oriented Programming (OOP) in Python
- Getters and Setters in Python
- Class Concepts: Object-Oriented Programming in Python
- Inheritance and Internals: Object-Oriented Programming in Python
- Design and Guidance: Object-Oriented Programming in Python
OOP is a big topic, and Real Python has more resources to help you expand your skill set. There’s even a learning path that’ll help you solidly grasp the fundamentals of OOP so that you can make your programs easier to write and maintain.
To continue your Python learning journey, check out the other Python Basics courses. You might also consider getting yourself a copy of Python Basics: A Practical Introduction to Python 3.
Congratulations, you made it to the end of the course! What’s your #1 takeaway or favorite thing you learned? How are you going to put your newfound skills to use? Leave a comment in the discussion section and let us know.
00:00 Congratulations on making it to the end of this Python Basics Exercises course. In this course, you practiced using classes, class attributes, instance attributes, instance methods, and also special methods that I’ve called dunder methods.
00:14 And you’ve also practiced working with f-strings. Additionally to training these specific skills, you’ve also trained more general skills, such as using code comments to help you get organized, break exercises into smaller tasks, using descriptive variable names, and also to test repeatedly to see whether the code that you’re writing actually does what you expect it to do. Nice work on all of this.
00:38 And if you want to continue training your OOP skills, I have additional resources for you. You can check out the article on Object-Oriented Programming in Python 3 that also has an associated video course.
00:49 You can look at the getters and setters and how to manage attributes in Python and Operator and Function Overloading in Custom Python Classes.
00:57 We also have a big article on Classes: The Power of Object-Oriented Programming and three video courses that are loosely based on the information in this article. So you can check out Class Concepts: Object-Oriented Programming in Python, Inheritance and Internals: OOP in Python, and Design and Guidance: OOP in Python. So as you can see, there are a lot of resources on OOP in our course catalog.
01:22 So if you want to continue training and learning more about this design pattern in programming, make sure to go to the search and find the article or tutorial that you’re interested in. And that’s it. Congratulations for making it to the end, and thanks for joining, and I hope to see you in another Python Basics Exercises course.
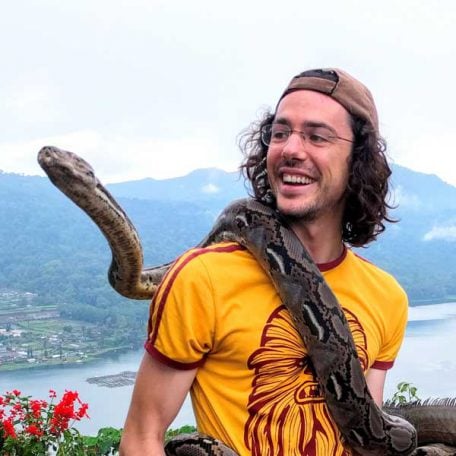
Martin Breuss RP Team on Nov. 22, 2023
@alphafox28js your code is a good attempt and works, but it doesn’t quite follow how OOP and classes work in Python.
If you define a new method with the same name as a previous method in the same class, then you’ll overwrite the previous method. There can always just be one.
Currently, there’s a lot of unnecessary duplication in your code. Think about how you would write your code if you had to instantiate 1000 cars? Try to refactor your code to remove duplication and update your variable names to reflect the generalization.
alphafox28js on Nov. 23, 2023
@Martin Breuss, I ended up coming up with this as an improved from the listed above.
#Improved Class of Car
class Car:
def __init__(self, color, mileage):
# Instance Attributes:
self.color = color #creates Attribute called color and assigns the value of color parameter to it.
self.mileage = mileage
def __str__(self):
return (f"The {self.color} Camero with Red Blood Stripes, has {self.mileage} miles on it.")
def drive(self, miles): # method on instance of car
self.mileage += miles # adds mileage to original int value passed in via self.mileage = mileage
#instantiation
Camero_0 = Car("Olive Green", 20000)
Camero_0.drive(0) #Add .drive(miles) to increment self.milage original int value
print(Camero_0)
Camero_1 = Car("Blue", 30000)
Camero_1.drive(777)
Camero_1.drive(250)
print(Camero_1)
while Camero_0.mileage < 100_000:
Camero_0.drive(5_000)
print(Camero_0)
while Camero_1.mileage < 150_000:
Camero_1.drive(7500)
print(Camero_1)
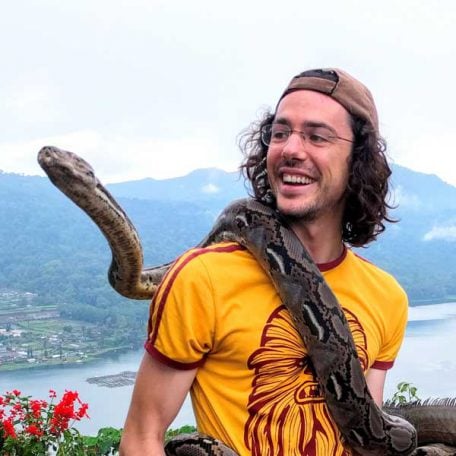
Martin Breuss RP Team on Dec. 12, 2023
@alphafox28js that’s a great update!
The only nitpick I have for you is that the variable names for your Car
objects should start with lowercase letters:
camero_0 = Car("Olive Green", 20000)
Starting with capital letters is conventionally reserved to classes—but not to variable names that hold instances of classes.
Become a Member to join the conversation.
alphafox28js on Nov. 19, 2023