How to Use the map() Function
In this lesson, you’ll learn how to use the map()
function in Python in order to apply a function to all of the elements of an iterable and output an iterator of items that are the result of that function being called on the items in the first iterator.
You’ll walk through a practical example of how to use the map()
function with the example data set that you’ve been working with in this course so far. You’ll know how to get a new iterable containing all of the information that you need from the old iterable, but you won’t modify the old iterable in the process!
00:00
What we’re going to do now is we’re going to use the map()
function to do some interesting stuff with it. Typically, you want to use the map()
function if you want to take something like this, like this list here, and you want to transform it into a different list. Basically, all it does—it takes a list of stuff, applies a function to each item, and it assembles a new list based on that. What we can do here, I thought—you know, it’s always a little bit hard to come up with good examples, but what I thought would be fun to do here—if we would actually take this list here and we would assemble a new list that
00:37
contained the names and ages of these scientists. So, we’re going to ignore most of these fields here and we’re going to focus on the name
and then some calculated property that’s based on the born
field and the birthdate.
00:53
I thought a good way to do this would be to use the map()
function.
00:59
Here again, we’re going to use the tuple()
function, first, to convert the output of the map()
function into a tuple. I’m going to show you what it looks like without the tuple()
call, but just so you get a proper output that’s actually more interesting.
01:16 And then, what we’re going to do,
01:18
we’re going to define this function that we want to map. Here, I’ll do it with a lambda, which is just a one-line function in Python that has a single—it can take arguments, and then it can… It has a single expression here and it will just automatically return the result of that expression. All right, so what are we going to do here? In this case, I’m just going to define a dictionary object, for lack of a better idea, and we’re going to plug in the .name
and we’re also going to create this new field here called 'age'
.
01:52 I’ll just calculate the age based on the birthdate of the person.
01:58 That way, we’ve defined our function that gets mapped across all of these items. Then, we just need to make sure our braces here—or, our parentheses are balanced.
02:14
Okay. Before I run this, let’s take a look at this from, like, a high-level perspective. We’re defining this thing here, names_and_ages
, and that’s going to be the result of mapping this function, here,
02:30
over the list of scientists. This is going to create a new tuple, actually, so, we can sort of treat this like a list. It’s going to create a new collection, or ordered collection that’s based on the scientists. For each Scientist
, it’s going to create a new dictionary here that holds all of these calculated properties. So, okay. I’m just going to show you what this looks like.
02:53 All right. Nothing happened, because we need to print this out and, actually, this looks pretty ugly, so let’s pretty-print it. Okay. What you can see here—and I’m hoping you’re starting to get the idea—this took the initial list and it transformed it into a new list that was
03:11 generated by mapping a function onto each of the original items.
03:18
So now, in this new list here, we don’t have all of the information we had previously. Now we have a name—well, we have the 'name'
, and we have the 'age'
. There’s one downside to doing it this way because I defined a dictionary here, so this—again—is, like, a mutable—this is a mutable data structure because we can reach in and modify these dictionary objects. So, if you want to do this better, you would probably want to define a new namedtuple
object, but I kind of wanted to do it in one expression here.
03:48
So, this really took the initial list and transformed it into this new list without destroying the original list, right? Which is kind of beautiful and which, again, shows you the power of functional programming, because we have this little map()
function call here, and it did all of this in one go and it was very declarative. We didn’t have to spell out the loop like, “Oh, for each x in scientists
do this and add it to a list.” I can all do it in one go, which is kind of neat.
Donna van Wyk on Sept. 24, 2019
Wow. I’ve been learning about python a lot of diff ways. Your “simple” explanation of a lambda here finally made it click. I am so excited to go thru all of the courses and really get somewhere.
agarwalamit081 on Aug. 1, 2020
By using the dictionary first and then converting to a tuple for the filtered results, we have now lost the original order of the filtered result. Is there a way to avoid this?
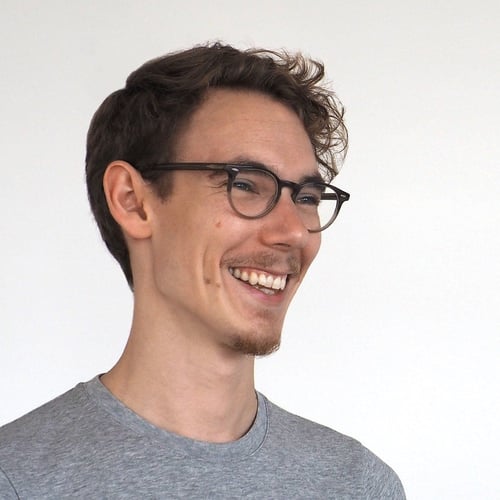
Dan Bader RP Team on Aug. 2, 2020
On Python 3.6 and above, dict
retains the original insertion order automatically. On older Python versions, including Python 2.x, you can use the OrderedDict
class from the standard library.
DroxyDopa on Oct. 23, 2020
Why isn’t pprint function working on my Python 3.8.5?
Become a Member to join the conversation.
Shay Elmualem on Aug. 3, 2019
This is great stuff Dan, thanks! I normally, out of habit, just go with the “manual” approach of iterating/looping over and just getting/saving the data I need, I really need to start using these built-ins more! very cool.