Manipulating Strings With String Methods
00:00 Strings come bundled with special functions—they’re called string methods—that you can use to work with and manipulate strings. There are numerous string methods available, as you’ll see. First, you get to focus on a handful of commonly used ones.
00:17 In this lesson, you’ll learn how to convert a string to uppercase or lowercase, how to remove whitespace from a string, and how to determine if a string begins or ends with certain characters. Okay, time to learn your first method.
00:34
The first one is going to work on converting the case of the string. That method is .lower()
. It’ll convert the string to all lowercase letters, taking any capital letters and turning them into lowercase.
00:47
So it looks something like this. If you add the string of "Jean-Luc Picard"
, and then you add .lower()
as a method, it’ll return all lowercase characters. The .
tells Python what follows is the name of a method, in this case the .lower()
method.
01:04
You’ll hear this referred to very often with string methods, using a .
at the beginning of their names, for example .lower()
or, as you’ll learn here in a second, .upper()
.
01:15
This makes it a little easier to differentiate functions that are string methods from built-in functions like print()
or type()
that you’ve learned earlier.
01:26
The methods will work on a string by itself by adding the .
and lower
. Don’t forget to call the method with the parentheses. They also work if you’ve assigned them to a variable.
01:42
So in this case, if I type name
and put the .
in and want to return the lowercase version of name
.
01:52
Okay, now that you’ve played with .lower()
, the next one you’re going to learn is .upper()
. So .upper()
converts a string to all uppercase letters.
02:03
If you take the same variable, name
, and apply the .upper()
method, you’ll see that it returns all capital letters. So just one more comparison between methods and functions that you saw previously: string methods will begin with a .
at the beginning of their names, and that’s how you’ll refer to them, with the leading “dot.” So, “dot lower” or “dot upper.” That’ll make it a little easier to differentiate functions that are string methods from built-in functions like print()
or len()
. When you compare them, aside from the different results of these functions, the important distinction is how they’re used.
02:41
These built-in functions are just called by themselves. So if you want to find the length of the name
string, you call len()
and put name
inside of it, or if you want to print name
, you would do the same thing vs how on the other hand, with .upper()
and .lower()
, they need to be used in conjunction with a string.
03:01 They don’t exist independently.
03:07 Whitespace is any character that is printed as blank space. This includes things like spaces, tabs, and even line feeds, which are special characters that move output to a newline.
03:18 Sometimes you need to remove whitespace from the beginning or end of a string. This is especially useful when working with strings that come from user input, something that someone’s typed in, which may sometimes include extra whitespace characters by accident.
03:32
There are three string methods that you can use to remove this whitespace from a string: .rstrip()
removes trailing spaces from the right side of the string, .lstrip()
removes preceding spaces from the left side of the string, and .strip()
removes whitespaces from both left and right sides, so anything at the beginning or at the end of the string would be removed.
03:55 Interestingly, it doesn’t remove spaces from the middle. Let’s try some of this out in the interactive window.
04:05
First, we need to create some whitespace, so I’ll make a new name
, put a couple spaces and a tab, and I’ll put my own name in again. You can use your own name. In this case, I’m going to add a couple spaces and a tab, and then \n
which is a line feed. Actually, I’ll add two of those line feeds with a couple spaces in between. So there’s a lot of different whitespace there. In fact, if you try out print()
with name, you’ll see all the white space, including the additional line feeds, the two of them. Okay, so what happens if you use .rstrip()
? It’s stripped away all the whitespace characters from the right side, including the line feeds. Again, name
’s still the same, so if I do name
and .lstrip()
, that will get rid of the whitespace at the front.
04:57
And if we use .strip()
, it gets rid of all the spaces on both sides, all the whitespace, be it tabs, spaces, or line feeds.
05:11 When you work with text, sometimes you need to determine if a given string starts or ends with certain characters. You can use two string methods to solve this problem.
05:21
The first is .startswith()
, and the other is .endswith()
. Let’s try it out in the interactive window.
05:30
Let’s say we have the string "Enterprise"
. We’ll create a variable called starship
and capitalize "Enterprise"
. If you use the method .startswith()
, and in this case, you need to, you can see it requesting what you’d like that prefix to be, and what if I put lowercase "en"
? Will that work? That returns False
.
05:51
You might have figured out why—because it starts with a capital "E"
, the fact that the .startswith()
method is actually case sensitive. To get this to be True
, you’d have to type the same thing, but start with a capital "En"
. That would return true.
06:06
And similarly, if you wanted to try out the other one, .endswith()
. In this case, let’s say you say, does it end with "rise"
? You’d get True
.
06:15
This, too, is case sensitive. The True
and False
values are not strings. They’re a special kind of data called a Boolean value.
06:25 You’ll learn more about Boolean values in a later lesson.
06:31
Do you remember talking about in a previous lesson, how strings are immutable? Most string methods alter a string like .upper()
and .lower()
, but they actually return copies of the original string with those modifications.
06:46
So if you aren’t careful, you might not recognize this, and it might introduce subtle bugs into your program. Try this one out. So put a name. This time, we’ll say "Francis"
. Okay, so again, if you type name
and put in the method .upper()
, you get all uppercase, but you might have noticed, I showed this a little earlier, too, how the original is not modified. Now to get it to change the variable name
, you would need to reassign it.
07:14
So that would look like this: name = name.upper()
. Now name
has been changed. That will override the original string that you assigned to name
by reassigning it.
07:31 You might have noticed that there are a lot of methods associated with strings. The methods that I’m showing in this lesson barely scratch the surface. IDLE can help you find these new string methods.
07:42
Let me show you how you can discover some of them. Let’s go back to our starship
example. If you start with the variable name, starship
, and then add the dot, a little box will pop up and start to show you some of the different ones that are available. If you type a letter, you’ll also get some of them. It’ll narrow down the results. In fact, if I start to spell upper
, you’ll see it highlighted there.
08:10 You can complete this by pressing Tab, and then you can finish by calling the method. This is a nice way that you can discover additional methods that are available.
08:26 Okay, so now that you’ve practiced a little bit trying to find some of those additional methods, here’s a quick review using IDLE. You can type the name of the variable that’s assigned to the string, and then you add a period, often spoken as “dot,” and then you wait.
08:41 Typing in a letter will narrow in some of the results, and pressing Tab will automatically fill in that method name if you’ve started to name it. And one note, this even works with variable names.
08:54
Try typing the first few letters of starship
and pressing Tab. If you haven’t defined any other names that share those first couple letters, IDLE completes the name starship
for you.
09:05 So Tab is a nice sort of auto-complete that you can use across IDLE.
09:12 Okay, time to review what you’ve learned. Here’s a couple exercises for you to try out. Write a program that converts the following strings to lowercase, then print each lowercase string on a separate line.
09:28 Try repeating exercise one, but convert each string to uppercase instead of lowercase. Write out a program that removes whitespace from the following strings, then print out the strings with all of the whitespace removed.
09:44
Write a program that prints out the result of .startswith("be")
on each of the following strings.
09:54
Using the same four strings as exercise 4, write a program that uses string methods to alter each string so that the .startswith("be")
returns True
for all of them. So again, write a program that uses other string methods to alter the strings so that .startswith("be")
returns True
for all of them.
s150042028 on Sept. 3, 2023
>>> animals = "Animals"
>>> badger = "Badber"
>>> honey = "Honey Bee"
>>> honey2 = "Honey Badger"
>>> print(animals.lower())
animals
>>> print(badger.lower())
badber
>>> print(honey.lower())
honey bee
>>> print(honey2.lower())
honey badger
That OK. I can’t insert the images.
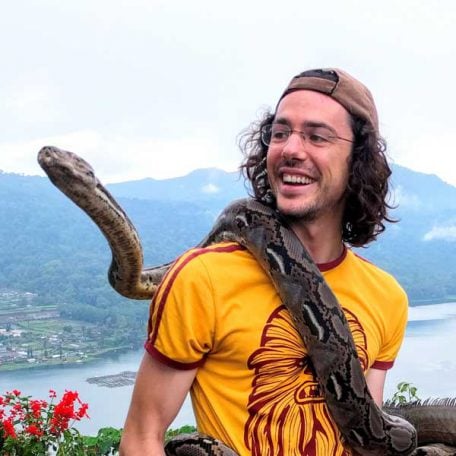
Martin Breuss RP Team on Sept. 4, 2023
@s150042028 that’s great and works to solve the first exercises. Also nice job formatting the code in your comment! :)
s150042028 on Sept. 9, 2023
animals = "Animals"
badger = "Badber"
honey = "Honey Bee"
honey2 = "Honey Badger"
print(animals.lower())
print(badger.lower())
print(honey.lower())
print(honey2.lower())
print(animals.upper())
print(badger.upper())
print(honey.upper())
print(honey2.upper())
string1 = " Filet Mignon"
string2 = "Brisket "
string3 = " Cheeseburger "
print(string1)
print(string1.lstrip())
print(string2.rstrip())
print(string3.strip())
string11 = "Becomes"
string12 = "becomes"
string13 = "BESR"
string14 = " bEautiful"
print(string11.startswith("be"))
print(string12.startswith("be"))
print(string13.startswith("be"))
print(string14.startswith("be"))
print(string11.lower().startswith("be"))
print(string12.lower().startswith("be"))
print(string13.lower().startswith("be"))
print(string14.lower().lstrip().startswith("be"))
I hope I’m doing well. I don’t know. Please rate my work, if it’s OK. Any suggestions or something wrong as elementary student.
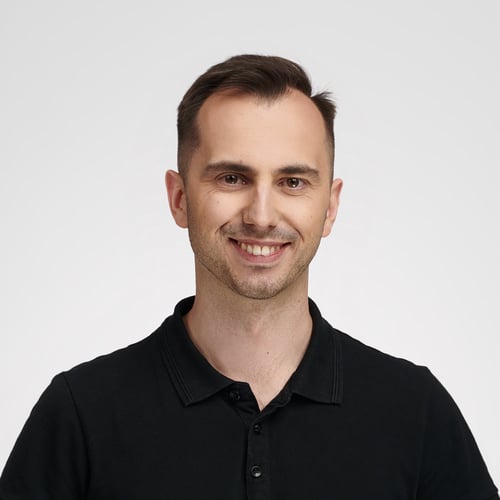
Bartosz Zaczyński RP Team on Sept. 11, 2023
@s150042028 There’s nothing wrong with your code, which works correctly. You convert strings to lowercase, convert other strings to uppercase, remove whitespace, and correctly check if your strings start with a certain sequence of characters. Overall, this looks like a good start 👍
Mr B on Sept. 27, 2023
GRRRR. The way I undrestood it was to create a list.. pets= “Animals, “Badger”, “Honey Bee”, “Honey Badger” and seperate the list[....]to print on seperate lines, along with convert to lower, print the lower-list, as seperated lines. then make upper, print upper seperated list.... or am I biting too much off? I cant figure out how to seperate the list??
Mr B on Sept. 27, 2023
OK, try this.. why.py
pets1 = "Animals"
pets2 = "Badger"
pets3 = "Honey Bee"
pets4 = "Honey Badger"
print (pets1.lower() )
print (pets2.lower() )
print (pets3.lower() )
print (pets4.lower() )
print (pets1.upper() )
print (pets2.upper() )
print (pets3.upper() )
print (pets4.upper() )
Mr B on Sept. 27, 2023
More_why.py
string1 = " Filet Mignon"
string2 = "Brisket "
string3 = " Cheseburger "
string1 = string1.strip()
string2 = string2.strip()
string3 = string3.strip()
print (string1)
print (string2)
print (string3)
And then there is Belogny.py
string1 = "Becomes"
string2 = "becomes"
string3 = "BEAR"
string4 = "bEautiful"
print()
print (string1)
print (string2)
print (string3)
print (string4)
print()
print ('Do the strings start with "be"?')
print ("string1 startswith be:", string1.startswith("be") )
print ("string2 startswith be:", string2.startswith("be") )
print ("string3 startswtih be:", string3.startswith("be") )
print ("string4 startswith be:", string4.startswith("be") )
print()
print ('Using .lower() to turn the strings to lowercase')
string1 = string1.lower()
string2 = string2.lower()
string3 = string3.lower()
string4 = string4.lower()
print()
print ('Do the strings start with "be"?')
print (string1)
print (string2)
print (string3)
print (string4)
print()
print ("string1 startswith be:", string1.startswith("be") )
print ("string2 startswith be:", string2.startswith("be") )
print ("string3 startswtih be:", string3.startswith("be") )
print ("string4 startswith be:", string4.startswith("be") )
print()
print('All the strings start with "be"')
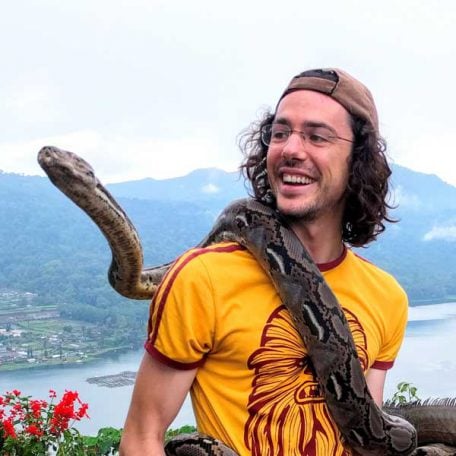
Martin Breuss RP Team on Sept. 29, 2023
Mr B you tackled the challenge like intended, nice work :)
There’s no need to create a list, you’ll learn about lists later on in the learning path.
I’m also in the process of recording an exercise course that walks you through these review exercises and challenges, so if you want to check back in a bit, then that’ll be available for this course as well.
Mr B on Sept. 30, 2023
Overachievers, they’re so distracted by semantics. I guess the ‘ADD’ kicked in and overly complicated things.
Become a Member to join the conversation.
Andres Rengifo on July 5, 2023
This might be one of the best explanation I have come across about string methods, great!