Working With Dictionary Values
For this lesson, you’ll need the following dictionary:
my_dog = {
"name": "Frieda",
"age": 5,
"nicknames": ["Fru-Fru", "Lady McNugget"],
"hungry": True,
}
Now you’re ready to work with dictionary values!
00:00
In the last lesson, you created your own dictionaries. Now I’ll show you how to access, add, change, and delete dictionary values. In this session, we’ll work with the my_dog
dictionary again.
00:13
If you have closed your session from the last window, pause this video and re-create the dictionary as you can see it right here. You can also find the code in the description below or in the materials we are adding to this video course. So when you type my_dog
, you should have the same output as you can see on the screen: 'name':
'Frieda', 'age': 5, 'nicknames':
with a list of 'Fru-Fru'
and 'Lady McNugget'
and also the key 'hungry'
with the value True
.
00:44 So every time you want to see the whole dictionary, you can just let Python output the dictionary for you. But if you don’t want to see the whole dictionary, then you can access the values directly.
00:59
Here you know that there is the key "name"
in the my_dog
dictionary. This means you can type my_dog
, square brackets ([]
), and then "name"
.
01:12
Don’t forget the quotes around name
because you are referring to a string.
01:18
So as you can see, here you can access the name directly and Python outputs "Frieda"
, which is the name of my dog. When you want to add a new key-value pair to the dictionary, then you can use a similar notation.
01:33
Let’s also add information about my dog’s breed to the dictionary. So again, it’s my_dog
,
01:42
then square brackets. This time, let’s put "breed"
as a string. This will be the key. And the value is also a string with the content "poodle"
because my dog is a poodle.
01:58
If you have a look at the my_dog
dictionary now, then you can see that 'breed': 'poodle'
was added at the end of the dictionary.
02:09 Oh, and by the way, prior to Python 3.6, the order of key-value pairs in a Python dictionary was unpredictable. In later versions, the order of the key-value pairs is guaranteed to match the order in which they were inserted.
02:23
So if you happen to following along with a Python version older than 3.6, then you’ll notice that the output of dictionaries in general will be different than what you’re seeing here. Okay, now let’s change a value in the my_dog
dictionary. Again, the syntax looks exactly the same except you’re using a key that already exists in the square brackets. So, currently, Frieda’s age is 5
.
02:57 Let’s say today is her birthday. Happy Birthday, Frieda!
03:04 Then you can change the value by writing
03:19
When we have a look at the complete dictionary again, then you see that the age is now 6
in the dictionary. This works because each key in a dictionary can be assigned only a single value.
03:33
That means when a key is given a new value, then Python overrides the old one. If you want to remove a key-value pair from a dictionary, you can use the del
keyword. Okay, so we accessed a value with a key, we created a new key-value pair, and we changed the value.
03:54
Now it’s time to show you how to remove a key-value pair from a dictionary. To remove a key-value pair, you can use the del
keyword. We can assume that my dog is always hungry, or at least that’s what she tells me.
04:10
So let’s remove this item from the my_dog
dictionary.
04:22
When we let Python output the my_dog
dictionary, then you can see that 'hungry': True
is now not part of the dictionary anymore. Oh, and one important note before we wrap up this lesson.
04:37
Don’t forget to actually add the square brackets with the key to the dictionary name if you want to delete a key-value pair. If you would only write del my_dog
, then you would delete the whole dictionary. This can be handy sometimes, but that’s not what we want to do right now. So again, with del my_dog
and the key "hungry"
in square brackets, we deleted the key-value pair for "hungry"
.
05:00 If you now try to access the item,
05:11
then you’ll get a KeyError
. The KeyError
is probably the most common error that you will encounter when working with dictionaries. In the next lesson, we’ll explore how to prevent running into this error by checking if a key in a dictionary exists first before accessing it.
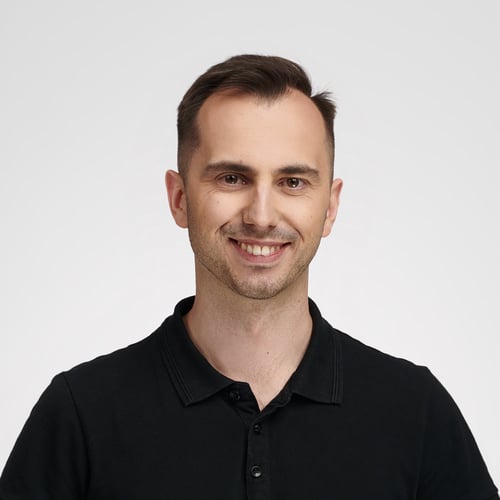
Bartosz Zaczyński RP Team on April 28, 2023
@stefaniabolota It sounds like you defined your variable my_dog
as a tuple rather than a dictionary. A dictionary literal uses curly braces as opposed to regualar parentheses:
>>> a_tuple = (1, 2, 3)
>>> a_dict = {1: 2, "key": 3}
ted750770 on Jan. 17, 2024
I am using Python 3.9.2.
I type, my_dog_dict = del my_dog_dict["hungry"]
I get an error, “Invalid syntax”.
Please tell me what my error is.
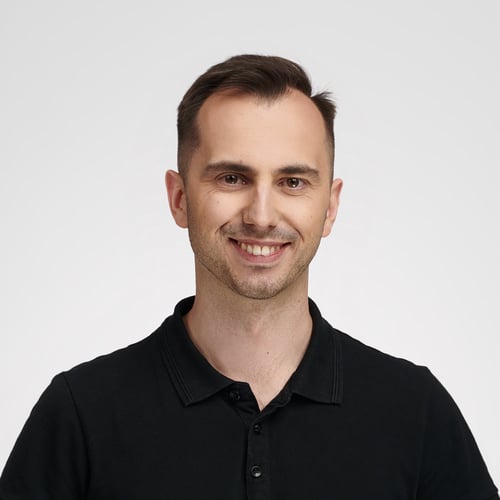
Bartosz Zaczyński RP Team on Jan. 17, 2024
@ted750770 In short, del
is a statement in Python, not an expression, which means you can’t use it on the right-hand side of the assignment operator (=
).
When you use the del
keyword, it doesn’t return any meaningful value that you could intercept and assign to a variable on the left. Instead, deleting a key-value pair from a dictionary works in place, so you’ll see the change by accessing your my_dog_dict
variable again:
>>> my_dog_dict = {"name": "Frieda", "hungry": True}
>>> del my_dog_dict["hungry"]
>>> my_dog_dict
{'name': 'Frieda'}
This is known as a side-effect using the programmer’s lingo.
Become a Member to join the conversation.
stefaniabolota on April 28, 2023
When I type
my_dog["name"]
, it shows me an error that is:Can you please let me know what it is? I also use IDLE. Thank you.