Creating the Flask Application
To learn more about the concepts covered in this lesson, you can check out:
00:00 Creating the Flask Application. In this section, you’ll learn how to create a Python Flask example application and run it locally. You’re going to initialize the project, install Flask, create the application, and run it on your computer.
00:16 You’ll also learn how to use Git to version your application’s code. The project initialization consists of creating a directory for your application, setting up a Python virtual environment where dependencies will be installed, and initializing the Git repository.
00:33 You don’t have to use a virtual environment or Git for local development, but they are very convenient and will make development and deployment to Heroku simpler. For more on virtual environments, check out this Real Python course.
00:48
Start by creating a new directory for the Python Flask example app. You can do it by running these commands seen on-screen. Here you create a realpython-flask-app
folder, and then change the current working directory to that folder.
01:05 Next you’ll create a Python virtual environment. Using a virtual environment allows you to manage your project’s dependencies without messing with system-level files shared by all applications.
01:17 You’ll see the commands needed to create and activate a virtual environment. The first line works on all platforms.
01:27 The command needed to activate the virtual environment depends on the platform that you’re on. Here’s the command for macOS and Linux,
01:40 and here’s the command for Windows.
01:48
These commands create a virtual environment named venv
and activate it so packages will be loaded and installed from this environment instead of using the system-level packages. The next step is to install Flask.
02:01
You can run the following command to install Flask using pip
.
02:16
This installs Flask version 2.0.3, the version you’ll be using throughout this course. Next, you need to create a requirements.txt
file listing the project’s dependencies.
02:29
You can use the pip freeze
command for this task, as seen on-screen.
02:39
You’ll use requirements.txt
when deploying the project to tell Heroku which packages must be installed to run your application’s code. Now the application directory should look as seen on-screen.
02:54
Next, you’ll create a small Flask application with a single route, /
(index), that returns the text "Hello World!"
when requested. To create a Flask application, you have to create a Flask object that represents your app and then associate views to routes.
03:10 Flask takes care of dispatching incoming requests to the correct view based on the request URL and the routes that you’ve defined. For small applications like the one you’re working on within this course, you can write all the code in a single file, organizing your project as seen on-screen.
03:27
app.py
contains the application’s code, where you create the app, and its views. Create app.py
and edit the contents as seen on-screen.
03:42
First, you import Flask
. After Flask
is imported, this code creates the object app
, which belongs to the Flask
class.
03:52
The view function index()
is linked to the main route using the @app.route
decorator. When the main route is requested, Flask will serve the request by calling index()
and using its returned value as the response.
04:08
There are different ways in which you can run this application. One of the most straightforward ways to launch a Flask app for local development is using the flask run
command from the terminal, as seen on-screen.
04:22
By default, Flask will run the application you defined in app.py
on port 5000
. While the application is running, go to the address seen on-screen using your web browser.
04:34
You’ll see a web page containing the message "Hello World!"
, as you see on-screen. During development, you’d normally want to reload your application automatically whenever you make a change to it. You can do this by setting an environment variable, FLASK_ENV
, to development
.
04:55 Here’s how to do this on macOS and Linux,
05:03 and here’s how to do this on Windows.
05:12
You can now run Flask again, and you will see that the environment has changed. When you set FLASK_ENV
to development
, Flask will monitor changes to the app files and reload the server whenever there’s a change. This way, you don’t need to manually stop and restart the app server after each modification. In this course, you are going to track the changes to your project’s files using Git, a popular version control system. You’ll need to install Git, and the method of installation will vary depending on which platform you’re running on. Follow the link on-screen and install Git for your operating system.
05:52
Once Git is installed, as a first step, you should create a Git repository for your project. You can achieve this by executing the following command in your project’s directory. This command initializes the repository that will be used to track the project’s files. The repository metadata is stored in a hidden directory named .git
.
06:16
Note that there are some folders that you shouldn’t include in the Git repository, such as the virtual environment and __pycache__/
. You can tell Git to ignore them by creating a file named .gitignore
and adding the folders to it, as seen on-screen.
06:37 Next, you see the commands on-screen that you use to add the files to the Git repository
06:46 and make a first commit.
06:53
After running these commands, Git will track changes to your application files, but it will ignore the venv/
and __pycache__/
folders.
07:03 Now the project directory should look as seen on-screen. If you want to learn more about Git and hosting your repository on GitHub, then check out this Real Python course.
07:16 You’re now ready to deploy your app using Heroku, and that’s what will be covered in the next section of the course.
Nexthazard on March 2, 2024
Windows User - “set Flask_ENV=development” didn’t work for me, had to create a .env file then add “FLASK_ENV=development” to it but to get it to work I had to do a pip install “pip install python-dotenv” (suggest running pip freeze > requirements.txt after to add it to requirements file) then everything worked.
jmflannery82 on July 2, 2024
when I try the “flask run” command, I get an error that says:
Error: Could not locate a Flask application. Use the ‘flask –app’ option, ‘FLASK_APP’ environment variable, or a ‘wsgi.py’ or ‘app.py’ file in the current directory.
After some googling, I found that I needed to run the command:
set FLASK_APP=app.py
However, this still didn’t help. Still getting the same error.
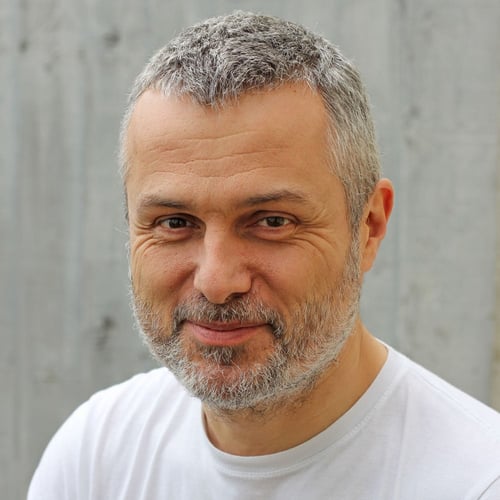
Darren Jones RP Team on July 2, 2024
I think you may be in the wrong directory when you’re running the flask run
command? I’ve just tried downloading the course materials (app.py) into an empty folder, and from that folder, flask run
is working for me.
If you run ls -al
(or dir
on Windows), what do you see for the contents of the directory you’re currently in?
Become a Member to join the conversation.
Nexthazard on March 2, 2024
ImportError: cannot import name ‘url_quote’ from ‘werkzeug.urls’
Solution: Change Werkzeug version to 2.2.2 in requirements file (Werkzeug==2.2.2)
Then run ‘pip install -r requirements.txt’