For more information on topics covered in this lesson, check out these resources:
Using Explicit Returns in Functions
00:00
Now let’s look at explicit return
statements. When a Python function executes an explicit return
statement, the function immediately ends its execution and sends the return value back to the calling environment. As previously mentioned, a return
statement begins with the keyword return
, followed by an optional return value. For example, in this simple function, the statement return 42
means the number 42
will be returned back to where the function was called.
00:34
Just a simple function with a single return
statement,
00:41 and that’s it! When I call this function,
00:48
I get the number 42
back. And since we are in the interpreter, that number is displayed. The return value for a function can be used in any expression, so I can save it to a variable or just use it directly from the function call.
01:08
Here I am saving it to the value num
, and there it is. But I can also do it with a calculation.
01:22
There I’m multiplying it by 2
.
01:27
And here I am adding 5
to it. And in each case, the number 42
is put in place of where the function call was in that expression, and then the rest of the expression is evaluated.
01:39
So I got 42
times 2
, 84
, and then 42
plus 5
, 47
. The operations have to make sense.
01:50
Since this function returns a number, its function call can only be used where a number would be valid. You can’t use a return
statement outside of a function.
02:03
It generates a SyntaxError
. So if I try just a return
statement, return 42
, there you can see at the bottom of the screen, my interpreter’s told me Syntax Error: ‘return’ outside function.
02:18
return
statements only makes sense inside the body of a function. And recall that methods—regular methods, class methods, static methods—are just functions within the context of a class or an object, so anything you’ve seen here about functions applies to methods as well.
02:41 Here’s another example. This function expects a list of integers as a parameter and returns a new list with only the even numbers from the original list.
02:54
This function uses a Python syntax called list comprehension. It’s a way to simplify a common for
/if
nested coding structure, which filters a list, looking for those elements meeting a specific condition.
03:11
Real Python has many resources for list comprehension, some of which are linked below. If we take a look at the function more closely, we see that it takes an argument, a list of numbers called numbers
.
03:27
We then look through the list one element at a time for those numbers which are divisible by 2
. If a number is divisible by 2
, then its result modulus 2
is 0
.
03:44
0
itself is considered a False
value, so if you want the condition after the word if
to be True
, you have to put not
in front of it.
03:56
This will build up a new list, selecting from numbers
those elements which have a remainder of 0
when divided by 2
.
04:08
That list is saved to the variable even_nums
, which in the next line is used as the return value, so the return value here will be that newly created list.
04:30
and then call get_even()
on a list of numbers,
04:36
since we’re in the interpreter, the return value will be displayed when the function finishes executing. We can see we get the numbers 2
, 4
, and 6
, that list created from the original list.
04:52
The return value can be any valid Python expression. We could simplify the get_even()
method by just returning the result from the list comprehension without saving it to a different variable name.
05:07 So I could remove that, and just return the list comprehension. Let’s save that,
05:22 and I’ll restart my environment so I can re-import it.
05:29
from evens import
our new get_even
. But again, give it a list of some numbers and it will provide for us a new list with just the even numbers.
05:48 Here’s another example where I can use Python’s sum and length functions to write a new function to find the mean of a collection of numbers. Just returning the expression, we’re going to take the sum divided by the length.
06:03 I won’t work through this one, but feel free to experiment with it on your own.
06:09 A common source of confusion is the difference between returning a value and outputting a value, and we’ll go into that next.
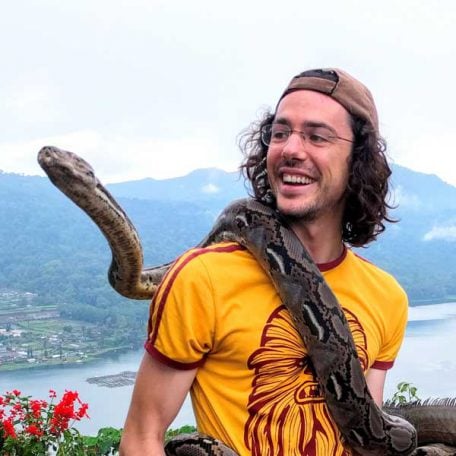
Martin Breuss RP Team on Aug. 30, 2021
Hi @j63811, there’s no newbie rubbishing going on here, and all questions are valid!
My thoughts on your observation is that it really depends on what are your preferences. There are no hard rules here, only soft ideas of what (maybe) a majority (?) of the Python community considers as “idiomatic” or “pythonic”. You might have heard these terms somewhere.
Some would argue that the falsy nature of the value 0
gives you the opportunity to shorten the statement by evaluating a non-boolean value in a boolean context. This approach and code length reduction might make it more readable for them:
if not number % 2:
# Your code
You might argue that this obfuscates the actual value of the modulo operation, where the result isn’t False
, but the number 0
. Therefore, you prefer to be explicit:
if number % 2 == 0:
# Your code
Both of these approaches are perfectly fine, and you should use the one that makes more sense to you. :)
There are always many ways to do the same thing in programming. It’s good to be clear and concise with your code, and make sure that you’ll still be able to understand what your intention were also months in the future. Hope that helps!
j63811 on Aug. 30, 2021
Thank you Martin great, when learning it’s difficult to tell if a trait is symbolic or traditional or just too clever for it’s own good. Just to be nudged into knowing that you are understanding but have preferences is very helpful
Become a Member to join the conversation.
j63811 on Aug. 29, 2021
Good tutorial and thank you, I am going to be newbie rubbished and sorry in advance but I just dont see how ‘If Not number % 2” is more explicit or immediate than ” If number % 2 == 0 ” what am I missing ?