Inheriting From Other Classes
00:00 Another way to create relationships between classes is to use a mechanism called inheritance. Inheritance can be a tricky concept to wrap your head around or understand when it might be useful in a real-life situation. That said, it is a handy tool—maybe not as common as composition, but it’s still handy. For instance, inheritance can be really helpful in creating variations in classes.
00:26 Imagine that you have a large class with many methods, and then it turns out you need another class, just a small variation on that class. It only differs in one single method.
00:39 Do you write the whole class again with just one different method? No. You can use inheritance to do that very cleanly. Inheritance also happens to be a favorite source of OOP interview questions, so it’s well worth being exposed to it.
00:57
For the example, you’re going to be working with this Doggo
class, which represents a dog. This Doggo
class has a class attribute, a constructor method, a special instance method, or a dunder method, and a normal method.
01:18
This is how you instantiate various instances of Doggo
with this current class structure.
01:26
Then you can choose any of these doggos and get them to speak, and they all behave in exactly the same way. That’s to be expected. But maybe you want to define different breeds of Doggo
, and you want that to be obvious from the class name. Well, inheritance gives you a way to do that.
01:46
Take a look at these class definitions. They rely on the existing definition of the Doggo
class, as you can see here. These are subclasses of the Doggo
class.
01:58
The way you define a subclass is you take the class
keyword, as you do with normal classes. You have the name—again, as you do with normal classes—but instead of going straight to the colon (:
) you add in some brackets (()
) as if it were a function.
02:14 Just a quick note on terminology here. When I say brackets, I mean round brackets or parentheses. If we come across square, curly, or angled brackets, I’ll explicitly say so. But if I just say brackets, I’m referring to round brackets or parentheses.
02:33
And you add in the parent class here. So this allows you to subclass Doggo
into various different classes. So we’ve got JackRussellTerrier
here, Dachshund
, Bulldog
.
02:45
Now these names will come up whenever you instantiate an instance from these classes. Note that we’re just leaving these empty for now. The default behavior of this will be that since its subclassing Doggo
, these classes are going to behave exactly the same as the Doggo
class.
03:04 Since you’re passing, you’re saying I’m not changing anything from the parent class.
03:12
So now you can instantiate your Doggo classes through their subclasses, which points to their breed. So now you can say JackRussellTerrier(
"Miles", 4)
years old, or jack
is a Bulldog
.
03:26
He’s called "Jack"
and he’s 3
.
03:31
Let’s take a look at that in action. Here you’ve got your Doggo
class loaded up. This will serve as the parent class. Note this code formatting.
03:41 This is so that it fits in the window without going off the edge. If you have two f-strings together, they will automatically concatenate. And the way you make sure that these are taken together is with the brackets, which is missing one here.
04:02 So these just act like one string …
04:10 like this, but as you can see, you have to scroll off the right edge of the screen. So …
04:22
this is equivalent. Anyway, say you wanted to make another type of class with slight variations, let’s just say with the name of the class for now. So we can say class
Labrador()`, and using the brackets, within that, we put in the parent class.
04:43 Then we have the colon, and for now, all we want to do is change the name of the class but keep everything else about the class the same. Control + S to save, F5 to run.
04:55
And that runs without any errors. So now we can create the dog called gandhi
, and we’ll say he’s a Labrador
. His name is "Gandhi"
, and he is an old dog, at 13
years old.
05:11
Note that you’re just using the same constructor as the Doggo
class for the Labrador
class because the Labrador
class inherits from the Doggo
class.
05:21
And since it’s not overriding or changing anything, we can just assume that everything within the Doggo
class is now also within the Labrador
class. So instantiating this is no problem.
05:34
And then we can say gandhi.name
, 'Ghandi'
. gandhi.age
, 13
. We can even look at the class attribute, 'Canis
familiaris'
. We can print(gandhi)
.
05:51
Gandhi is 13 years old
, as per the special dunder method. And we can say gandhi.speak("buf")
,
06:02
and 'Gandhi says buf'
. So there you are. That is the most basic form of inheritance. You can also check instances. So isinstance()
, let’s see, gandhi
is an instance of Labrador
.
06:22
We expect that to be True
because it’s the direct instance of that class. But you can also check
06:31
if gandhi
is an instance of Doggo
. What do you expect to see here?
06:38
It’s True
because a Labrador
is an instance of a Doggo
because it’s within that inheritance chain. And you could subclass Labrador
again into say, LabradorRetriever
, and the LabradorRetriever
would still be an instance of Doggo
because it shares that inheritance.
07:03 That’s an introduction to inheritance. You’ve seen how you can extend classes. That means having a parent class, which you then subclass. Note that you may not need inheritance. Composition is easier to get your head around, and for a lot of cases, it’s more flexible than inheritance.
07:21 But there are some situations when you need slight variations where inheritance can be very useful. Just don’t feel the need to use it everywhere. And that’s inheritance.
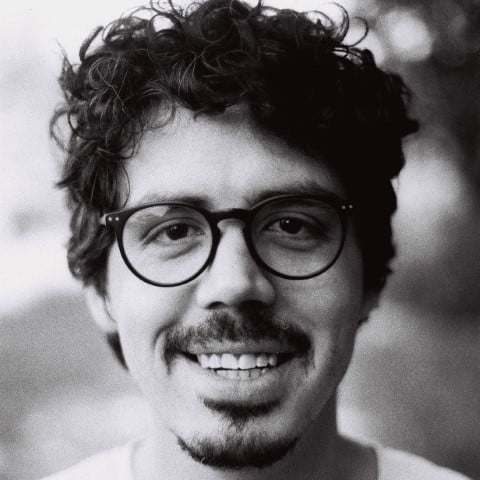
Ian RP Team on Aug. 31, 2023
Ha! It is a bit of an odd name, but my old rescue dog came with that name :) He was a very good boy!
Vamsi B on April 26, 2024
Great content , bad example , though not a big fan of “Gandhi” , as an Indian to see him represented as a Dog is a bit humiliating , he sure must have given you some pain in the past.
Shashi kiran on July 25, 2024
Great course but using the name “Gandhi” is not good. That is not politically correct.
Become a Member to join the conversation.
akshaya3193 on Aug. 24, 2023
Gandhi?! odd name for a dog, don’t you think?