Integers
00:00 Let’s get started looking at integers.
00:05 Integers—what is an integer? Well, an integer is a whole number, the kind you started out counting out when you first learned about numbers at school. In Python 3, an integer is any length up to the memory limit of the computer, so you probably won’t run out of integers that you can use.
00:25 The default is in decimal—base 10—but as you’ll see, you’ll be able to define them and view them in different bases. You’re going to see how to create integers and different ways you can work with them in the following videos.
00:42 Throughout these workshops, our coding will be done using bpython, which is a version of the Python REPL that uses docstrings and color coding to make understanding code more simple. With that housekeeping out of the way, let’s look at ints.
00:57
As you’ve already seen, an int
is a whole number, and they can be created easily as seen here—creating a variable a
with a value of 1
and b
with a value of 10
.
01:11
We can see that a
has a value of 1
, and b
has a value of 10
. It’s possible to do simple arithmetic, such as a + b
, seen here, or a - b
. And the results are as we would expect. As mentioned previously, the default for these numbers is decimal, or base 10, but it’s possible to use other bases.
01:37
We can have them in binary, hexadecimal, or octal. If we want to define one in binary, here we can see we’re defining c
, and we start off with 0b
and then put in the binary digits that make the number up.
01:53
And now we’ve defined c
, and if we hit c
and Enter, the value—as you can see—is 343
in decimal. This is a nice, quick, easy way to convert binary numbers to decimal.
02:09
Here, one can be defined in octal, again using a similar pattern, but 0
and then o
, for octal, and then putting some octal digits.
02:18
And again, d
equates to 153802
in decimal. And e
will be defined in hexadecimal using 0x
before the digits which make it up—in this case, ac4d
.
02:32
And that equates to 44109
in decimal. Now sometimes, you’ll be in a situation where you’ve got a string which is a number, but you need to equate it to an int
.
02:47
Here I’m going to define my_num
as a string of '1235'
. If you try and do some addition with that, my_num + b
, you can see it doesn’t work.
03:02
We get a TypeError
and you can see that it’s trying to concatenate the string and b
. So, it’s actually not doing what you’d think at all, and certainly not the addition we were looking for.
03:16
So what’s needed is to convert my_num
into an integer to allow maths to be performed on it. That’s done using the keyword int()
, and inside we’ll put the original variable my_num
, and you can see that now equates to the number 1235
, whereas my_num
is the string with the quotes enclosing it.
03:40
And there you can see the int
doesn’t have any quotes.
03:48
Now, we can define my_num_2
as the int()
with a value of my_num
, and now it’s possible to do the maths on it. + b
gives us the value of 1245
.
04:05
As you’ll see with all of the variables in this course, it’s possible to inspect the type of them using type()
. So here, type()
and then we put any of our variables in, and we can see that they are the <class 'int'>
, which is for integer variables.
04:21
And helpfully, you can see that the reported <class 'int'>
is the same as the keyword used to cast a variable into that type.
04:33 You’ve already seen how to define an integer using a base of binary, oct, or hexadecimal, but it’s also possible to perform the translation the other way around.
04:45
Now you’re going to see that in action using the my_num_2
variable, in converting it to different bases. First, it’s possible to create a binary representation of it using the bin()
keyword, and now you have the binary representation of my_num_2
. Using oct()
gives us the octal representation of my_num_2
.
05:08
And using hex()
gives us the hexadecimal version of that number. Remember, it’s still the same number—we’re just representing it in different bases.
05:19 You can see that these are three easy ways to represent the same number in binary, octal, or hexadecimal. And that covers integers!
Cory on April 23, 2020
May sound stupid, but why does bin(), oct(), hex() return a string? Does it have to deal with underlying programming of python seeing alphanumerics as a string? I apologize I like knowing how things work.
NICKS on Feb. 28, 2021
There aren’t any integer hex, octal, or binary objects. There are only alternative methods of creating integer objects.
The hex, octal, or binary are just string representation of the underlying integer objects. The link below explains it in a very clearly - mail.python.org/pipermail/tutor/2008-August/063967.html
avinashk2 on March 29, 2021
How to convert bin(27)
to base 10 int
since bin gives us string?
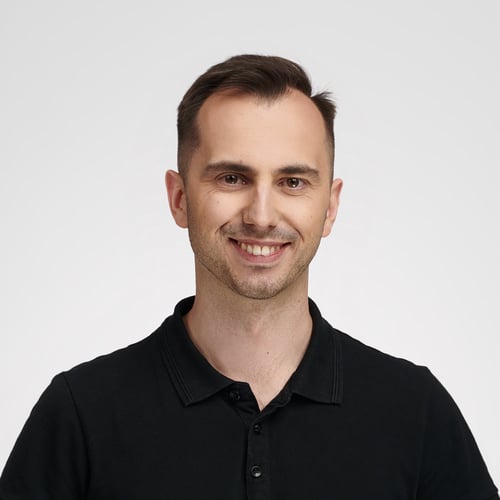
Bartosz Zaczyński RP Team on March 29, 2021
@avinashk2 If you call the built-in int()
function with two parameters, the first one is expected to be a string with digits while the second one is the base of the system, for example:
>>> int("11011", 2)
27
You can take the output of bin(27)
, drop the 0b
prefix, and pass it back to the int()
function:
>>> bin(27)
'0b11011'
>>> bin(27)[2:]
'11011'
>>> int(bin(27)[2:], 2)
27
david hodson on July 24, 2023
so i am can one just used the online content, i really do not have the patents to read through the book any suggestions
Become a Member to join the conversation.
emalfiza on Jan. 24, 2020
I had researched and dived to so many resources for the fundamentals of Pyhton, this is the first amazing one that I come across. Till now I didnt know we could get the Bin, Oct and Hex in python numbers… wow