Python time for Performance Measurement
00:00
In this last lesson, I’m going to show you a couple of useful functions that the Python time
module provides you for working with the performance of your code, measuring it and modifying it as necessary.
00:13
The two functions that I’ll be showing you are as follows. time.perf_counter()
, which is essentially a super precise little stopwatch, and just like a stopwatch, the perf_counter
doesn’t actually give you a real time, per se.
00:29 It doesn’t give you the time of day that it is, but what it does do is gives you a super precise time in floating-point seconds that you can then use to measure small increments of time.
00:40 So just like a stopwatch, you might click the start and then see that 10.5 seconds have passed, but you don’t know anything about the real time of day it is.
00:49
You don’t know whether those 10 seconds were from 3:00 PM to 3:00 PM and 10 seconds or midnight to midnight and 10 seconds. So that’s what perf_counter()
does, and then sleep()
allows you to pause the execution of a thread of a Python program for the specified number of seconds.
01:05 And so this is really useful for things like rate limiting, and any application where you need things to happen over sustained intervals of time rather than as fast as possible.
01:16 Let’s look in the REPL and see how these work in practice.
01:20
What I want to show you first is just the bare output of perf_counter()
. And as you can see, there’s a nanoseconds version as well.
01:29
This time output is obviously not the same as time.time()
, so it doesn’t represent the time since the epoch. What it represents instead is the very precise time since a recent fixed point, which means that it’s useless to determine the date, but what it’s really useful for is to say something like a = time.perf_counter()
, and then shortly afterward, you could say b = time.perf_counter()
, and then you can say b - a
and get a very precise account of the time delta
02:02 between those things. So it’s used for measurements between recent intervals, instead of being used as a way to reckon from some always-unchanging point.
02:13
What you should use this for is, instead, short distances between two things. You can see this in action, how it might be useful for functions by saying something like def test()
.
02:24
And then what you can do there is you can start at the beginning of the function, you can start a perf_counter
, then you can do some kind of unexciting work or very exciting work, depending on what you’re doing.
02:37
I’ll just do, maybe, 10 million times x = x + 1
. And then at the end, you can just return the end perf_counter
minus the start.
02:48 Then when you call it, it’ll hang for a little bit and then it’ll tell you that this took exactly, or not perfectly exactly, but very, very close to precisely, this amount of time: 0.73995 seconds.
03:01 So that’s really useful because there are a lot of applications where you need, really, precision timing. You know, science, engineering, all those sorts of things you require the time that is this precise.
03:11
So that’s really useful. Another function that’s useful is time.sleep()
. And it does exactly what you would think it does: it makes the program essentially go to sleep for however many seconds you pass in, and that just means it’s not doing anything for this time.
03:25 So if I pass it in 5, you can count down from 5 and it’ll just essentially do nothing for that time. Why is this useful? Well, there are a lot of occasions where it’s useful to do nothing for specified times, right? So think about rate limiting, for example.
03:41 If you’re querying some kind of server, you probably don’t want to always be querying that server. You want to give some gaps in there to allow your program to do other things or simply to allow the server to be able to give you that information and not have its resources constantly tied up by giving you back the information you’re requesting. So that’s one example, but there are many, many others where sleeping is actually something quite useful.
04:06
So I want to define one more test function, which is just test_sleep
, and I’ll say, let’s give it a parameter called x
, which will just be the number of seconds.
04:15
And then I’m actually gonna use perf_counter()
again, just to make sure that this thing is sleeping for the right amount of time. So you can say time.sleep(x)
and then just return, again, the perf_counter
minus the start.
04:29
And so you can run that and let’s give it, maybe, 5 seconds and as you can see, it will sleep for that amount of time and then it will return the perf_counter
, which, as you can see, is pretty much infinitesimally close to 5 seconds.
04:43
So some of that variation might just be the time it takes to call perf_counter
or other system factors that make this run a little bit longer than exactly 5 seconds. But as you can see, it’s vanishingly small, the actual difference.
04:56
So that’s how time.sleep()
works. It’s really simple, but it can be useful in a lot of surprising ways. I can’t tell you the number of times where I’ve had an application where I’ve said, “Oh, why is this performing so badly?” Well, it’s because it’s querying some server and I’m not giving it enough time to, kind of, react. Sleep can be very helpful in unexpected ways, so it’s good to know.
05:16
These are just a couple of the interesting functions that the time
module offers. I thought that they would be kind of fun and might be useful in your own code.
05:23 So take a look at the rest of the module’s documentation, if you’re looking for more interesting ways to interact with time and dates. Next up is the conclusion.
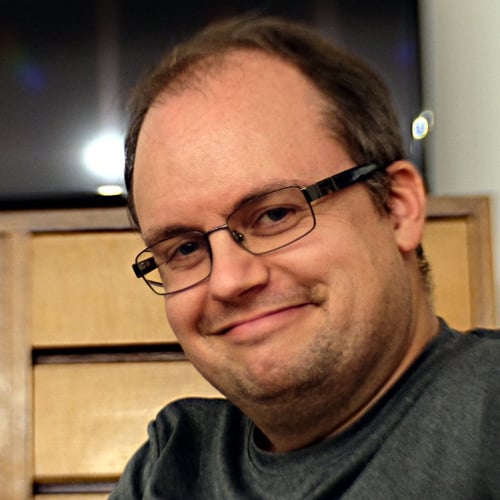
Geir Arne Hjelle RP Team on Aug. 11, 2020
@vincentstclair The reference point (i.e. time represented by 0) is undefined for perf_counter()
: docs.python.org/3/library/time.html?highlight=perf_counter#time.perf_counter
This means that perf_counter()
can not be used to figure out the time of day (you can use time.time()
for that, although the datetime
library is usually a better option), but it’s very useful for measuring time intervals.
We have some more information about the different timer functions in Python Timers: realpython.com/python-timer/#using-alternative-python-timer-functions
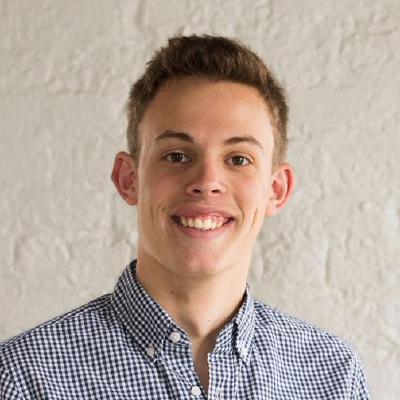
Liam Pulsifer RP Team on Aug. 11, 2020
Well said, @Geir Arne Hjelle! A salient detail from the docs is that perf_counter()
uses the most precise possible clock on your system, so the reference point can’t be defined in a system-independent way.
Vasanth Baskaran on Aug. 24, 2021
This course served the purpose for my today’s need. Wonderful course to get to know about core of time module. Thanks @Liam Pulsifer
Become a Member to join the conversation.
vincentstclair on Aug. 11, 2020
What does the number of seconds from the bare output of perf_counter() represent?
You:
Me:
What is this measuring, and how is it measuring it? What fixed point is it measuring from?