Time Zones
00:00 In this lesson, I’m going to cover a very important point in Python time, and in fact, in just how the world represents time as a whole, which is time zones. You might’ve been thinking during the last lesson, you know, all of this is great, but how do you deal with the fact that the whole world has many different time zones, all of which might have to have different representations of time, in Python as well? Luckily that’s what this lesson is here for.
00:25 So to understand time zones in Python, the first thing to understand is the concept of the UTC. And the UTC is a little strange because UTC actually stands for Coordinated Universal Time, but this is really a compromise between the English abbreviation, which would have been CUT, and the French abbreviation, which would have been TUC for Temps Universel Coordonné.
00:50 And I apologize to anyone who speaks French watching this video because I’m sure that was horrifically mispronounced. But regardless, so the English and the French would have had two conflicting abbreviations and they were the two main parties developing this concept, and so they decided to compromise by having an abbreviation that didn’t make sense with either time. So the UTC is a reference time zone, and it’s what allows pretty much all of the world’s time to really mesh smoothly with all of the different time zones that are around the world, because it’s like the epoch, but for time zones.
01:23 So the idea is, you have one fixed reference time zone, the UTC, which is kind of an imaginary time zone, in that it’s solely a reference. It’s not built to be, like, a real time zone.
01:35 And then other times zones, like the actual time zones of the world, are all defined via offset from UTC. So my time zone that I’m currently speaking to you from is EDT, Eastern Daylight Time, in the United States.
01:48 And so that’s at an offset of -4.0 from UTC, four hours behind the UTC.
01:55 Whereas the GMT, which is the Greenwich Mean Time, that’s the time zone at UTC plus 0.0, so that’s essentially the real time zone, which matches up with the UTC.
02:08
As I mentioned in the last lesson, there are several time
module functions that deal explicitly with time zones, and the two that I’m going to examine in-depth in this lesson, over in the Python REPL are gmtime
and localtime
.
02:21
And both of these take an optional seconds parameter, and if you don’t pass that parameter to either of these functions, it’ll just pass in the time.time()
, so they’ll default to the current time.
02:32
So the gmtime()
returns the Greenwich Mean Time, or essentially in line with the UTC time, as a struct_time
object, whereas localtime()
returns the local time. And the object that’s returned, the struct_time
, which I’m going to talk more about in the next lesson, also includes access to the time zone field and time GMT offset fields.
02:55 Those are pretty useful to kind of learn a little bit more about what time zone you’re in, et cetera. So let’s take a look at those over in the REPL.
03:03
So to work with time zones, with the Python time
module, as usual, you need to import time
,
03:09
and then the first example that I gave in the last lesson was time.gmtime
with a parameter of 0 seconds to give you the epoch, and as you can see that still works just as it did a second ago, but I think it’s instructive to take a look at the time.localtime
, 0 seconds from the epoch.
03:28
And as you can see, the epoch for the local time that I find myself in is not the same as the gmtime
. In fact, it’s defined in reference to that UTC epoch of January 1st, 1970, at midnight.
03:43 So the local epoch, you might call it, for the Eastern Standard Time, which is during that time of the year, what the local time is, it’s Eastern Standard rather than Eastern Daylight, as it is now in April, as you can see, it’s five hours behind.
03:59
So it’s December 31st, 1969, and 19 hours into the day, as opposed to 24, which is what this is. And so, as you can see, the time.localtime
, you can also use the parameters that I mentioned in the slide just a second ago, you can get the time zone, which is Eastern Standard Time because it’s in late December.
04:21
And then you can also get the tm_gmtoff
, I believe is what it’s called? Yes, exactly. And as you can see, it’s -18000 seconds from the epoch.
04:34 And so again, if you divide -18000 divided by 60 seconds in a minute times
04:41 60 minutes in an hour, then you get that it’s exactly five hours behind Greenwich Mean Time.
04:49
So of course, if I did, though, if I said just time.localtime()
right now, which is the same as passing it with time.time()
as the parameter, then I get: the year is 2020, the month is April, the day is the 14th, and it’s about 9 minutes after noon.
05:07
But if I do time.gmtime()
,
05:11 then I end up with the hour being 16, so it’s four hours ahead because at the moment I’m actually in
05:20
EDT, Eastern Daylight Time. So as you can see, all of this really starts to get complicated. And that’s what the main lesson that I want to provide you in this lesson about time zones is just that time zones are complex. As you can see, this has a tm_isdst
that’s 1
, whereas it wasn’t daylight savings time, simply because of the time of the year when I did time.localtime(0).tm_zone
, that’s Eastern Standard Time.
05:47 And so there’s a lot of complexity here, and what I really want to encourage you to do is try your best to understand this quite well, UTC and how the different time zones relate to that, but then, once you feel like you have a really good understanding, rely on a really well-documented and well-supported library to do all of that work for you.
06:05
The datetime
library is a fantastic library for working with dates and times, frameworks like Django will do a lot of the work of dealing with different user times for you already, so you don’t need to worry about that stuff. So that’s, I think, the main lesson that I want to provide in this short intro to time zones in Python is not necessarily that you need to be accounting for all of this in your own code, but that you need to understand why it’s necessary to use well-supported, Python or otherwise, libraries that already do a lot of this work for you. In the next lesson, I’ll take you through these data structures that I’ve been talking about and showing you, but I haven’t really explained in full detail yet.
06:45
So data structures like the struct_time
, floats, and tuples to represent Python time.
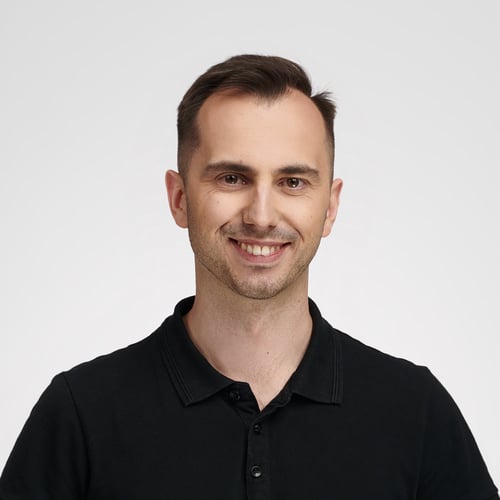
Bartosz Zaczyński RP Team on May 18, 2021
@sreesankar15207 To get a UNIX timestamp from a struct_time
tuple representing your local time, use the time.mktime()
function:
>>> import time
>>> time.mktime(time.localtime(0))
0.0
However, if you wanted to convert a UTC tuple, then use the calendar
module:
>>> import calendar
>>> calendar.timegm(time.gmtime(0))
0
sreesankar15207 on May 18, 2021
Sir, if I wanted to represent a specific date like my birthday. What should I do
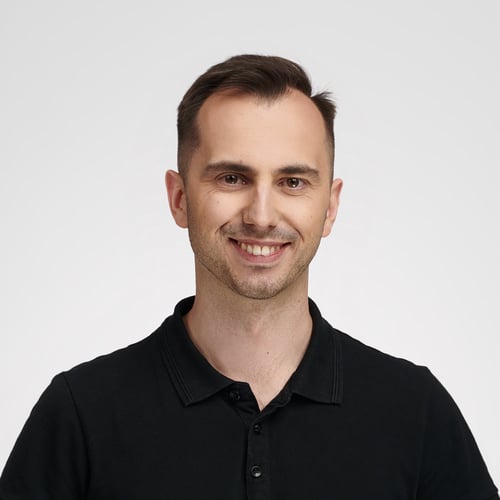
Bartosz Zaczyński RP Team on May 18, 2021
@sreesankar15207 The time
module is suitable for timestamps. However, date and time are better represented with the datetime
module:
>>> from datetime import date
>>> birthdate = date(1972, 12, 31)
>>> birthdate
datetime.date(1972, 12, 31)
sreesankar15207 on May 18, 2021
Thank you, Bartosz Zaczyński.
“Happy Pythoning”
Become a Member to join the conversation.
sreesankar15207 on May 18, 2021
Sir is there any way to change struct_time object to int so that I can perform calculations on that