Virtual Environment and FastAPI Setup
00:00 Before creating a REST API with FastAPI, it’s a good idea to create a new Python virtual environment. I’m in Visual Studio Code on Windows, but you can follow along on macOS or even Linux, or you can use your own editor, but Visual Studio Code has great Python support.
00:19 Press Control + ` to open the integrated terminal in Visual Studio Code, create a new directory for the demo project,
00:31
and then run Python -m venv .env
to create a new virtual environment. Depending on how Python is installed on your machine, you may be able to invoke Python itself.
00:45
The -m
option invokes a module named venv
that will create a new virtual environment in a directory called .env
, but you can name it whatever you like. After the virtual environment is finished, open the project directory in Visual Studio, and this can be done by executing this command.
01:07 In the project directory, Visual Studio will restart. Next press Control + Shift + P, or Command + Shift + P on macOS, to open the command pallet.
01:18 The Python extension provides support for virtual environments with the Python: Select Interpreter command. This will look for Python virtual environments—including those created by Conda, pipenv, and Poetry—and prompt you to select one.
01:33
Next, open a new integrated terminal, and VS Code will automatically activate the virtual environment for you. At the command line, install the FastAPI package using pip
.
01:49
Create a new Python file called main.py
in the project directory.
01:56
From the fastapi
module, import the FastAPI
class. Next, create an instance of the FastAPI
class. This will be the FastAPI app, which you’ll run later.
02:10 Now you need to create a function to handle an HTTP request to the API.
02:17 Notice that you’re returning a dictionary, but recall in the last lesson that data returned from a REST API is usually JSON. If you’ve worked with the JSON module in the Python standard library, you know that marshaling data between Python dictionaries and JSON objects is a cinch.
02:35
The two are almost syntactically identical. FastAPI will translate this data from a dictionary to JSON for you and return it in an HTTP response with the appropriate status code, 200
for OK in this case.
02:52
To map the function to an endpoint, use the @get
decorator from the FastAPI app, The @get
decorator accepts a path. Now, any request using the .get()
method or verb to the root of the API will be handled by the root()
function.
03:10
One more thing: recall that FastAPI supports async. That’s easy to add. Simply use the async
keyword before the def
keyword, and your function is now async.
03:25 Because FastAPI supports async, the API needs to be run on a server that supports async using the ASGI protocol. You may have heard of WSGI, or Web Server Gateway Interface.
03:39 For example, the package for Flask implements a WSGI server you could use to run Flask applications. ASGI is Async Server Gateway Interface, and you can install the uvicorn package and use it to serve your API.
03:58
To use uvicorn to serve your API, you can import it into main.py
and invoke the server in the entry point. This would be the only choice if you were using something like Replit to develop your API. However, it’s easier to run it from the command line.
04:15
This will tell uvicorn to run an ASGI app named app
in the main module, listen on all IP addresses on port 8000, and to automatically reload the application if the code changes.
04:32
If you navigate to http://localhost:8000
in a browser, you’ll see the JSON returned by the root()
function. Congratulations! You’ve created your first REST API using FastAPI. In the next lesson, you’ll learn how to use FastAPI’s interactive documentation.
sethcstenzel on April 12, 2022
I found out this was a known issue with using beta version 3.10.0b3. After checking which version you were using I installed 3.10.0 and this resolved the issue. Seems it was a known issue: github.com/samuelcolvin/pydantic/issues/3300
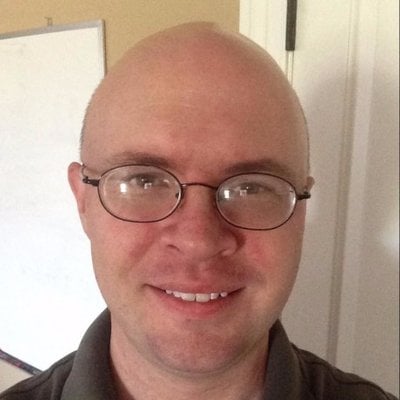
Douglas Starnes on April 13, 2022
@sethcstenzel
You can use Python back to 3.6. The course uses 3.9 and I’ve been using 3.10 without any issues. If you are working from the Repl online, it uses 3.8 and I have not had any problems. I try to avoid using betas when possible.
Become a Member to join the conversation.
sethcstenzel on April 12, 2022
Hmm, trying to follow this tutorial I am getting the following error when running uvicorn directly, and via import:
Any ideas?