In this video, you’re going to learn about the Django admin interface and how you can use it to conveniently interact with your database. Django comes with a few things built in from the get-go. In your settings.py
file, there were apps that came pre-installed. One of them is the admin
app.
Using the Django Admin Interface
00:00 Hey! In this video, we’re going to talk about the Django admin interface and how it allows us to conveniently interact with our database.
00:11
So as you know, Django comes built-in with a couple of things from the get-go. We’ve seen that—inside of our settings file—we saw that there’s a lot of apps that come already installed. One of them is the admin
app, and we haven’t seen this one yet, but we’ve encountered it before.
00:28
We’ve seen it here in INSTALLED_APPS
, and in our main urls.py
file there’s this endpoint called 'admin/'
that takes us somewhere.
00:38
Now, you already understand what’s happening here. So in this case, it’s going to some other URL configuration, but you can see that typing in /admin
should be taking us to somewhere.
00:48
Let’s give this a try. If instead of /projects
I type /admin
, let’s see where we go to. See? There’s like a whole nicely-styled page that’s just sitting there, that is part of our project that we didn’t even know about.
01:04
It’s called Django administration. So, how would we be able to log in here? We can’t yet, because we haven’t made this superuser that we need to be able to log into our Django admin
app.
01:18 So, what we need to do first is we need to create a superuser. I’m going to head over to the command line and do that right away.
01:27
With an open terminal window, I’m going to say python manage.py
, as nearly always, createsuperuser
. That’s the command that we need to—you guessed it—create a superuser.
01:41 So when I run this, it asks me for a username—I’m going to put in my name here. Email address—I’m just going to leave that blank. And then pass in the password—“Pass in the password.” All right. Repeat.
02:03 You’ve got to make passwords that actually match, type two times the same thing. And then it works, there you go. Our superuser was created successfully.
02:12 And now I’m able to log into this admin interface. Let’s go ahead and check it out.
02:20 I’m putting in my credentials. Let’s see if I can type my password correctly this time. It looks good. Okay. So, I’ve entered the Django administration in here and we can see, like, it looks like we’re probably able to do some things here: Add, Change, Users—what do we have in here? Let’s take a look. Interesting, so this is the user that I just created, and then there’s another superuser that I created earlier on.
02:49 So, there’s currently two users. And this looks very much like a table. So, what we’re interested in, actually, is not the groups and users, though. We’re interested in the projects, so that we would be able to add projects, edit projects, and delete projects from a convenient admin interface in here.
03:08
Django makes that actually very easy. Some things we need to do, and the first thing is we need to register our models in the admin interface. For that, we’re going to open up a file that we haven’t looked at yet inside of our project. That’s admin.py
.
03:28
We don’t really have anything in here at the moment, but Django gives us a nice prompt, again: Register your models here.
So, it’s talking about my models.
03:37
I want to have the Project
model, so I will have to import it. Let’s start off with that.
03:51 Then, to register it, what I need to type is
03:58
admin.site.register
and then pass in the name of my model that I just imported. And that’s really all. It’s just these two lines of code, and this already allows me to have access to my models inside of the Django interface. Let’s take a look.
04:18 When I reload this page,
04:22
my projects turn up here and I can take a look at them. I have my Project object (1)
and (2)
. Cool! And everything is in here, right?
04:33 So I can go ahead and edit directly in here. I can call this…
04:45 and then go ahead and SAVE. I’ll open up a new window so that we can see the changes right away.
05:03 And there you go! The title changed simply by typing it in here, which makes it very easy. Let’s go ahead and try and add a project. Title is going to be…
05:25
We need an image. Some suggestions of some that I did before, project/img/daily.png
. We have this one inside of our static/
files already—there it is—which means I’ll be able to link to it. I SAVE this.
05:45
It just has the number 5
here because I previously added and deleted some. It just keeps counting forward, but that shouldn’t be a problem for us.
05:56
Let’s take a look. It correctly renders! And here it is with a new image. Can I also click in here? I can! Great. And as you can see, because we’re using the id
, or the primary key, this is also number 5
, up here. Number 3
, and number 4
were created, but then deleted, but the database didn’t update the IDs.
06:17
So, it’s just going to keep counting upwards. For you, probably this is going to be number 3
.
06:27
Cool! So, let’s go ahead and try the other things that I just mentioned. I can also delete an object in here. Let’s get rid of this one. So I can say, Project object (2)
, Delete, and Go.
06:41 Yes, I’m sure, let’s get rid of it. Heading over to our app again, there it is! It’s gone. So as you can see, this Django administration is an awesome tool for interacting and updating your app, and this already comes built-in with Django.
06:57
So, the only thing you need to do is create your project and make sure that the admin
app is installed, register your models. And then you’re able to very, very conveniently interact with your database, add projects, remove projects, or edit projects—the text in there, the image, the technology, whatever you want.
07:16 So, that’s a great way of keeping your site easily up-to-date. With this, we’re at the end of this course. So, in the next video, we’re going to do a quick recap of what we learned in this section, and then I have a couple of suggestions for you in how you can still improve this project to make it even more awesome than it already is.
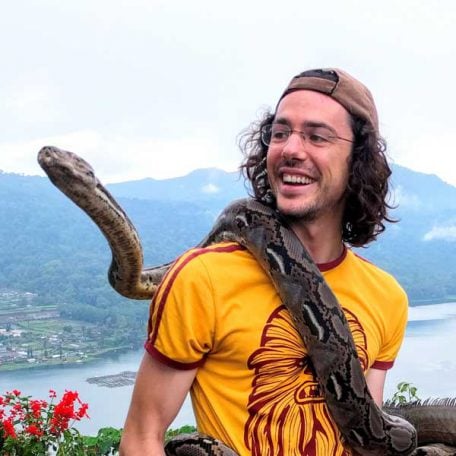
Martin Breuss RP Team on Oct. 22, 2019
Awesome tips! Thanks for posting these @Gascowin.
rolandgarceau on Jan. 24, 2020
What else needs to be done with the str() to get the rows to actually output the title?
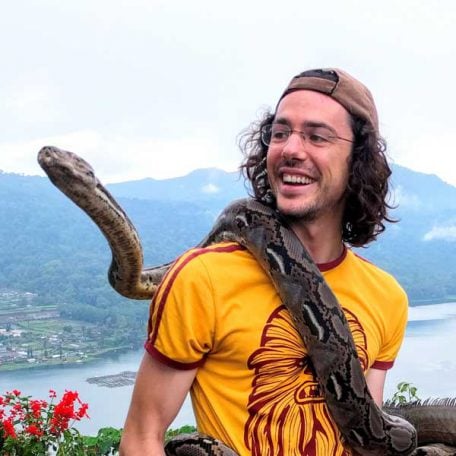
Martin Breuss RP Team on Jan. 24, 2020
Just what @Gascowin suggested. Make sure you’re defining the __str__()
method @rolandgarceau. If you add the following to your model it will output the project’s title:
class Project(models.Model):
# your model definition goes here
def __str__(self):
return self.title
You will also need to register the model in your admin interface, by importing it in admin.py
and then adding one line of code to register it:
from .models import Project
admin.site.register(Project)
That should do the trick! :) Read more about all the stuff you can do with Django’s admin interface in the official docs on The Django admin site
rolandgarceau on Jan. 27, 2020
What else needs to be done with the __str__()
to get the rows to actually output the title? I have started the blog and have the admin page displaying Categorys (somehow) under the blue highlighted blog. It appears to be the three names of the class definitions with an ‘s’ appended at the end, but I have yet to find what code is doing this. Has anyone figured that out yet?
rolandgarceau on Jan. 27, 2020
I just found that there may be only one admin.py file to use for this customization, as adding one in projects and then one in blog has irregular results in the admin page.
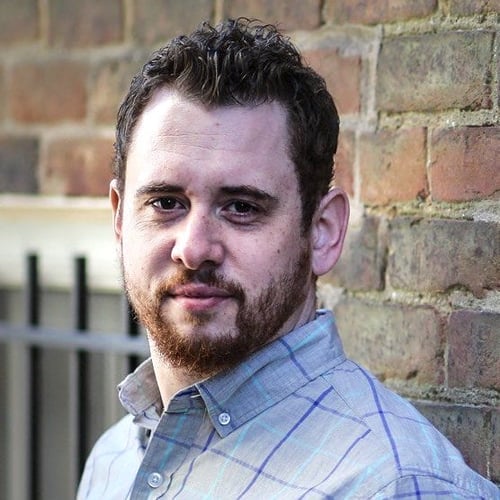
Ricky White RP Team on Jan. 27, 2020
@rolandgarceau To output the title in str()
you need to write:
def __str__(self):
return self.title
It appears to be the three names of the class definitions with an ‘s’ appended at the end, but I have yet to find what code is doing this.
Which three classes were you referencing here? Could you be more specific, please?
Mark R Baker on March 4, 2020
I have this weird problem: whenever I try to go to /admin, the server quits. The app works fine otherwise, but when I try to go to admin, it quits. Any ideas why? Mark
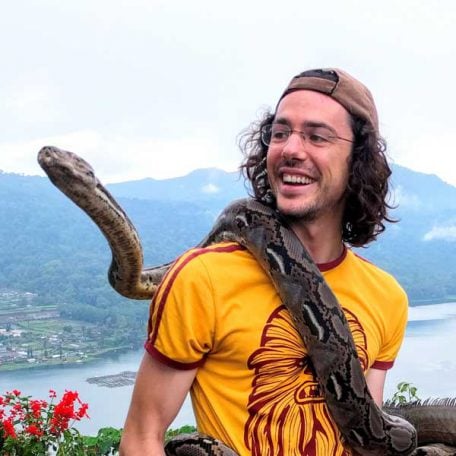
Martin Breuss RP Team on March 5, 2020
Hi @Mark R Baker! Check your terminal output where you are running the development server. It probably shows you an error message there that will be able to point you forward.
It is likely that you have some syntax error somewhere in your admin.py
file of your app, since that is the one you might have worked with.
When the development server quits only on a certain page, it is likely some code error in a file that would generate that specific page, and your terminal can tell you more.
Django’s front-end can’t display the error message in that case, since the program runs into an error before it can even build the application.
Mark R Baker on March 5, 2020
Hi Martin! Thanks for the quick response and for the great course. I am learning a ton. As per your frequent mentions, the first thing I did when this happened was scour for error messages. There were no error messages in the console. It would just behave as though I had press control + C. I will check the admin.py file for an error, but it seems unlikely, because after I encountered this problem, I moved the project to my iMac and it works perfectly there. I was running it on a windows 10 laptop before that. I just checked that machine and, indeed, there was nothing in admin.py. So that solves that. Thanks, Mark
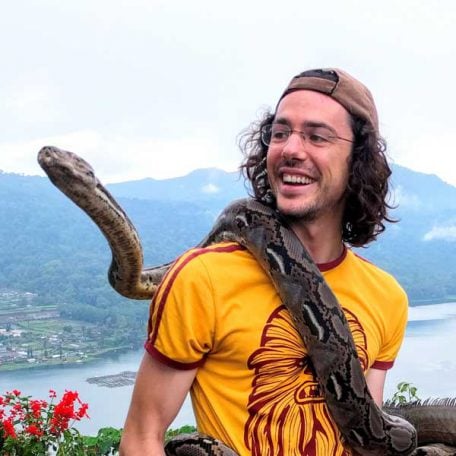
Martin Breuss RP Team on March 8, 2020
Awesome, glad you figured it out! Well done investigating 🙂👏
Mark R Baker on March 9, 2020
Well, Martin, actually, I cannot get the admin feature to work on my windows 10 ASUS laptop. It works fine on my iMac, but the same thing keeps happening on the laptop. localhost:8000/projects/ works just fine, but if I type admin, I get no error message; the server just stops running. I have checked what is written in projects/admin.py; it seems correct:
from django.contrib import admin
from projects.models import Project
# Register your models here.
admin.site.register(Project)
And it is definitely installed in settings.py:
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
# my apps
'projects',
]
Could it be that there is no admin.py file outside of the projects folder? Any help would be much appreciated. Mark
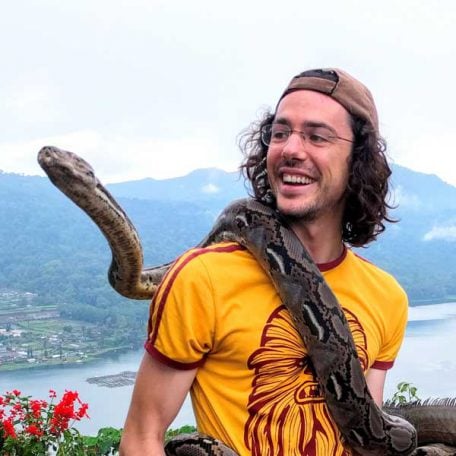
Martin Breuss RP Team on March 9, 2020
Hm… Ok, that does sound strange if the exact same code is working on your Mac but not on your Windows computer. 🤔
Check #1: Exact Same Code?
Did you clone the project that works on your Mac on your Windows machine, just to make 💯% sure that it’s the same code?
Check #2: URL Routing
Even though that shouldn’t be it if /admin
works on Mac and it’s the exact same code, can you still take another look in urls.py
of your management app?
It should have the admin URL registered there like so:
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
# and your include() goes here
]
Check #3: Django Installation
Maybe maybe something went wrong in the unfathomable depths of bits and bytes and a piece of code that you didn’t write is not working as expected. Usually that’s from accidentally changing or deleting something, or otherwise from a mysterious OS-level interaction that only the most enlightened of us can perceive…
So we gotta do the old-timer PC-technician-thingy and “turn it off and on again”, which in your case means:
- Make sure you’re inside your venv
- Uninstall Django
- Install Django again
Surprisingly often that fixes issues without anyone ever finding out what the problem really was 🙄
If that also doesn’t solve it and your server still quits, then it’s time for a:
Open-Source Rabbit Hole Deep Dive 🕳↙️🐇
Django is Open-Source, so here are a couple of steps that you can take to explore further.
Disclaimer: Be warned that if you accept this challenge, it might lead you down unexplored paths and is likely not gonna have a direct effect on solving this specific issue. If this is fun for you, however, you might just come out on the other end with a much better understanding of Django in general. If there is another end, that is 😅
- Package Code: You’ve installed Django as a package, which means you can find the source code in the package directory of your virtual environment. A one-liner bash snippet that will tell you where the Django source directory is located is:
python -c "import django; print(django.__path__)"
-
Namespaces & Folder Structure: From your code in
settings.py
you can see thatdjango.contrib.admin
is the app where your admin functionality lives. You can find it by following the namespaces. These are essentially folders inside thedjango
directory that you found with the previous command. Inspect the admin app and you’ll see that it is just another Django app with a similar structure to the ones you were building here. Fine, there’s a lot more code, but it’s essentially the same ideas here. -
The GitHub Repo: Finally, the code in that folder should be the same as the one you can find on Django’s official GitHub repo. If it isn’t then you either got different versions going or something got changed between your install and when you’re viewing the files. Now, that should not be the case after your uninstall-reinstall from earlier on, but who ever really knows
¯\_(ツ)_/¯
As mentioned in the disclaimer, I don’t know whether this rabbit-hole-dive will bring you any closer to solving your issue with Django on Windows. Honestly, if Check #1-3 didn’t do it, then this deep-dive is probably just a diversion tactic, lol 😜 Hopefully it can be educational, though!
I’m gonna ping some folks in the team that have more experience working with Python on Windows than me, and maybe they have some additional input on the matter. Please do report back whether any of the checks did the trick, and, if you want, also the degree of lost you got on the rabbit hole deep dive on a scale from 1-10 🤓
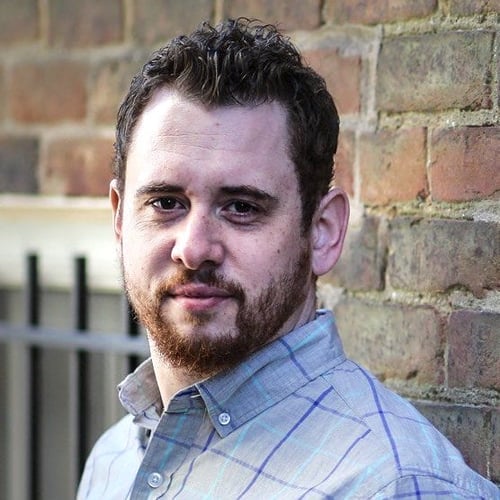
Ricky White RP Team on March 9, 2020
Hi Mark. I develop on Windows so I can help get it fixed. It would be helpful to see what you terminal is showing when it is running then when it stops itself. Can you copy and paste it into a comment. Also, not that is should matter, what are you using for your virtual environment (pip and venv, or pipenv, pyenv, or other)?
Mark R Baker on March 9, 2020
Thanks for your willingness to help, Ricky. Here is the command prompt log:
(.env) C:\Users\mrbak\python_code\django-portfolio>python manage.py runserver
Watching for file changes with StatReloader
Performing system checks...
System check identified no issues (0 silenced).
March 09, 2020 - 10:39:29
Django version 3.0.4, using settings 'portfolio.settings'
Starting development server at http://127.0.0.1:8000/
Quit the server with CTRL-BREAK.
[09/Mar/2020 10:39:37] "GET /projects/ HTTP/1.1" 200 4508
[09/Mar/2020 10:39:40] "GET /projects/1 HTTP/1.1" 200 1670
[09/Mar/2020 10:39:42] "GET /projects/ HTTP/1.1" 200 4508
[09/Mar/2020 10:39:47] "GET /projects/5 HTTP/1.1" 200 1776
[09/Mar/2020 10:39:49] "GET /projects/ HTTP/1.1" 200 4508
(.env) C:\Users\mrbak\python_code\django-portfolio>
I am using venv. Mark
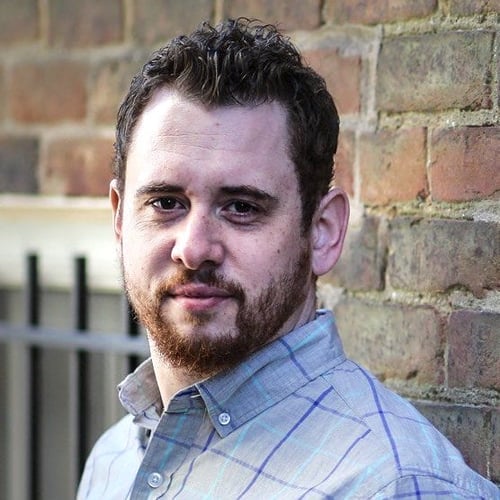
Ricky White RP Team on March 10, 2020
Hi Mark. I see it’s not even registering the GET
request for admin/
.
Is your code in a git repo I can look at it to see if I can recreate this error?
Mark R Baker on March 10, 2020
Thanks for taking a look, Ricky. Here is the git repo: github.com/mrbaker1917/django-portfolio.git
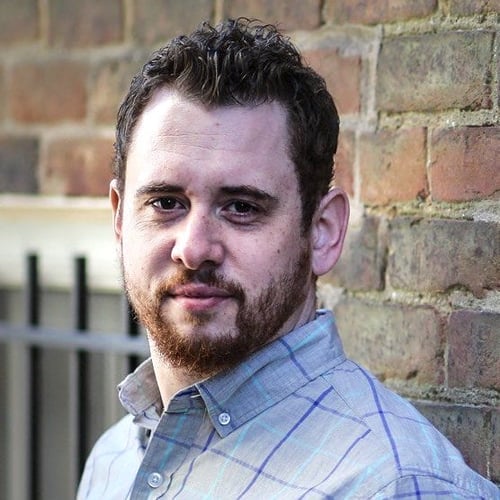
Ricky White RP Team on March 11, 2020
Hi Mark. I ran your project in a clean .env
that I made, and it worked fine. I was able to navigate to 127.0.0.1:8000/admin
and was presented with the login page. I see you have two environments in your repo. A .env
and venv
. I would delete both of those entirely and start a fresh. I would also advise that you not commit your environments to your git repos. So create yourself a .gitignore
file and add your .env
and database to it (you shouldn’t commit your database either.) You will also need to create a requirements.txt
to make your life easier for when you make your new environment. I hope all that makes sense. If not let me know and I can point you to some resources for further reading.
Mark R Baker on March 11, 2020
Thanks, Ricky! I did not realize one can delete the virtual environments without removing code that would make the app not work. So, I can simply delete those folders and redo the virtual environment step?
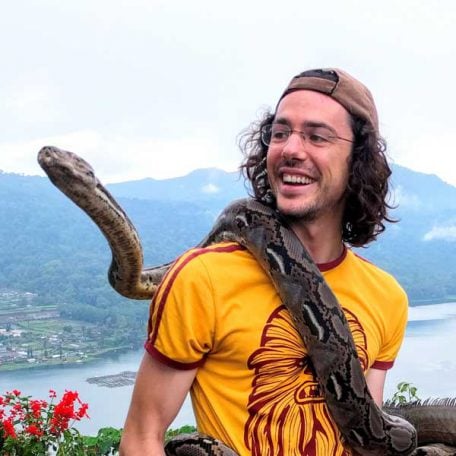
Martin Breuss RP Team on March 12, 2020
Hi @Mark! Yes, you can delete and re-create the virtual environment any time. That’s one of the beauties of compartmentalizing your development environment with virtual environments.
Give it a go and let us know how it went!
Mark R Baker on March 13, 2020
OK, so I deleted the django-portfolio from my windows laptop, cloned the repo from github, deleted the venv, set up a new one. Projects portfolio works fine, but when I try to access localhost:8000/admin/, I get the same problem as before:
(.env) C:\Users\mrbak\python_code\django-portfolio>python manage.py runserver
Watching for file changes with StatReloader
Performing system checks...
System check identified no issues (0 silenced).
March 12, 2020 - 17:03:59
Django version 3.0.3, using settings 'portfolio.settings'
Starting development server at http://127.0.0.1:8000/
Quit the server with CTRL-BREAK.
[12/Mar/2020 17:04:18] "GET /projects/ HTTP/1.1" 200 4508
[12/Mar/2020 17:04:21] "GET /projects/3 HTTP/1.1" 200 1776
[12/Mar/2020 17:04:23] "GET /projects/ HTTP/1.1" 200 4508
(.env) C:\Users\mrbak\python_code\django-portfolio>
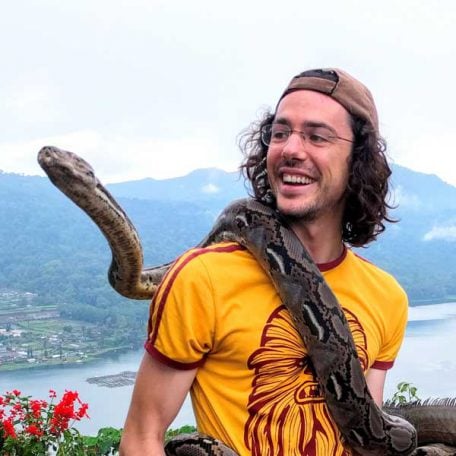
Martin Breuss RP Team on March 13, 2020
Hi Mark! Just to double-check, you:
- Exited your virtual environment with typing
deactivate
- Deleted the whole project folder (including your virtual environment folder called
.env
) from your Windows PC - Cloned the working project from GitHub into a new folder called
django-portfolio
- Created a new virtual environment and called it
.env
- Activated the virtual environment with
source .env/bin/activate
- Installed your dependencies with
pip install -r requirements.txt
(making sure you’re in an activated virtual environment by checking that the prompt shows its name, like it does in your code snippet above) - Ran the development server with
python manage.py runserver
You were (again) able to access the /projects
endpoint normally, but when you went to localhost:8000/admin
, the server quit without giving you any message or throwing an Exception in the command line. All you see after navigating to the admin URL is the CLI output you posted above, right?
Could you try these exact steps one more time, just to make sure that it really is a more mysterious problem and so we can rule out an issue with your virtual environment? Let’s see where this takes us!
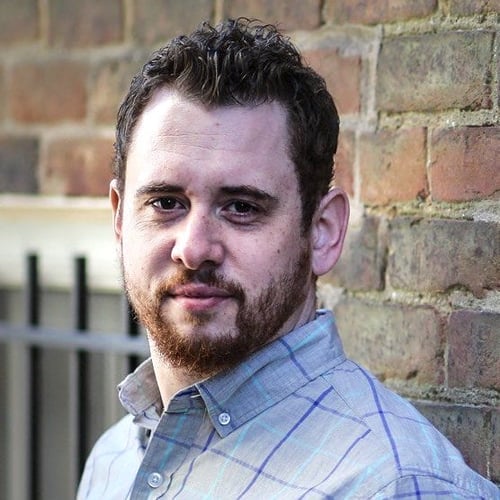
Ricky White RP Team on March 13, 2020
Mark. Do as Martin said above then we’ll assess. The only amendment I’d make, is as you’re on Windows, in 5.
you activate your virtual environment with .env\Scripts\activate.bat
.
Mark R Baker on March 13, 2020
Hello Martin and Ricky! You are too kind. I tried all these steps again. What happened: after I redid all this, admin worked for a few seconds, but when I tried to login, it quit as before:
(.env) C:\Users\mrbak\python_code\django-portfolio\django-portfolio>python manage.py runserver
Watching for file changes with StatReloader
Performing system checks...
System check identified no issues (0 silenced).
March 13, 2020 - 16:02:58
Django version 3.0.4, using settings 'portfolio.settings'
Starting development server at http://127.0.0.1:8000/
Quit the server with CTRL-BREAK.
[13/Mar/2020 16:03:23] "GET /projects/ HTTP/1.1" 200 4501
[13/Mar/2020 16:03:23] "GET /static/projects/img/testproject.png HTTP/1.1" 200 155219
[13/Mar/2020 16:03:23] "GET /static/projects/img/daily.png HTTP/1.1" 200 157031
[13/Mar/2020 16:03:23] "GET /static/projects/img/todo.png HTTP/1.1" 200 105814
[13/Mar/2020 16:03:26] "GET /projects/1 HTTP/1.1" 200 1748
[13/Mar/2020 16:03:28] "GET /projects/ HTTP/1.1" 200 4501
[13/Mar/2020 16:03:30] "GET /projects/2 HTTP/1.1" 200 1686
[13/Mar/2020 16:03:32] "GET /projects/ HTTP/1.1" 200 4501
[13/Mar/2020 16:03:34] "GET /projects/3 HTTP/1.1" 200 1785
[13/Mar/2020 16:03:36] "GET /projects/ HTTP/1.1" 200 4501
[13/Mar/2020 16:04:52] "GET /admin/ HTTP/1.1" 302 0
[13/Mar/2020 16:04:52] "GET /admin/login/?next=/admin/ HTTP/1.1" 200 1913
[13/Mar/2020 16:04:52] "GET /static/admin/css/base.css HTTP/1.1" 200 16378
[13/Mar/2020 16:04:52] "GET /static/admin/css/login.css HTTP/1.1" 200 1233
[13/Mar/2020 16:04:52] "GET /static/admin/css/responsive.css HTTP/1.1" 200 18052
[13/Mar/2020 16:04:52] "GET /static/admin/css/fonts.css HTTP/1.1" 200 423
[13/Mar/2020 16:04:52] "GET /static/admin/fonts/Roboto-Regular-webfont.woff HTTP/1.1" 200 85876
[13/Mar/2020 16:04:52] "GET /static/admin/fonts/Roboto-Light-webfont.woff HTTP/1.1" 200 85692
[13/Mar/2020 16:05:22] "POST /admin/login/?next=/admin/ HTTP/1.1" 302 0
(.env) C:\Users\mrbak\python_code\django-portfolio\django-portfolio>
And then, all further tries do the same thing as before. Server shuts down as soon as I try to access localhost:8000/admin/ Maybe I will just have to do all python/django stuff on the iMac. My son will be disappointed, as he prefers the iMac for games. Thanks again for all your help. Cheers, Mark
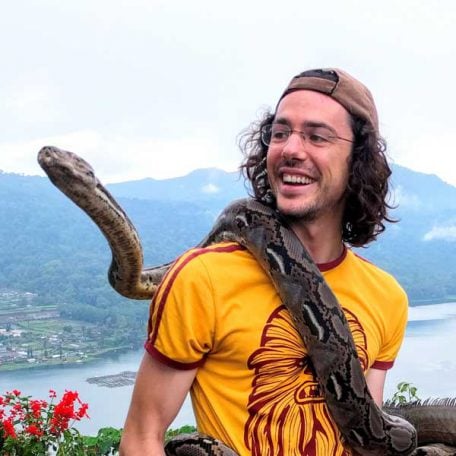
Martin Breuss RP Team on March 14, 2020
That’s interesting. It shows that it initially accesses the resources from your admin app to load the interface, but then breaks after the POST request, which is, from what you wrote, after submitting your login information and attempting to redirect you to the admin portal.
Another quick question: Have you created a superuser beforehand with:
python manage.py createsuperuser
You server shouldn’t quit also if you hadn’t made a superuser yet, but I’m just wondering.
Any ideas @Ricky how we could keep Mark’s son playing iMac games? 👾
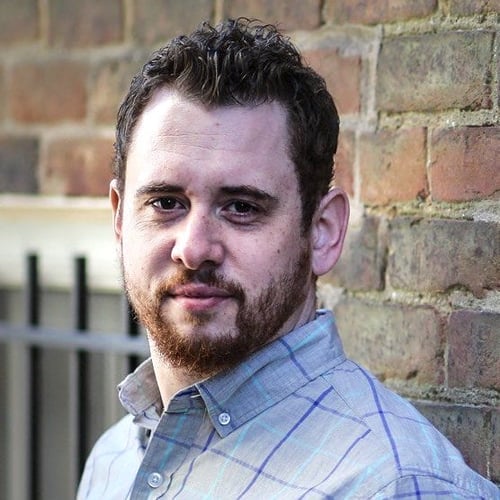
Ricky White RP Team on March 14, 2020
My mind went to the same as yours, Martin. There is a problem with the POST request. I’d be tempted to delete the sqlite.db
and start a fresh, making sure to create a superuser
and migrate
etc. I’ve done all my Django development on Windows, and never seen this.
Mark R Baker on March 14, 2020
The github version seems to already have the superuser I created earlier, so I tried to login with that userid/password:
C:\Users\mrbak\python_code\django-portfolio\django-portfolio>python manage.py createsuperuser
Username (leave blank to use 'mrbak'): mrbaker1917
Error: That username is already taken.
Should I create a new one? Thanks for all your help. Mark
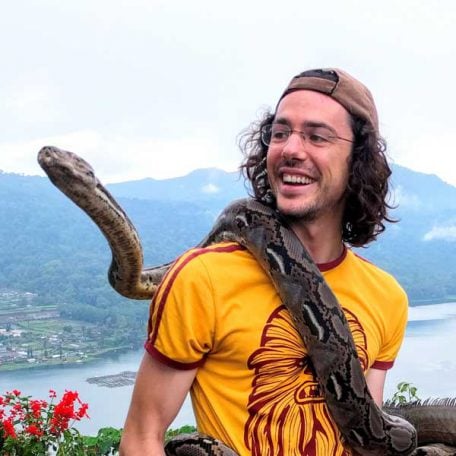
Martin Breuss RP Team on March 15, 2020
As Ricky mentioned, I’d also suggest to delete your db.sqlite3
file, then run your migration commands again:
python manage.py makemigrations
python manage.py migrate
And, after that, create a new superuser:
python manage.py createsuperuser
Something might be off in your database file, so deleting it and re-creating it might do the fix.
Databases and Version Control
Generally you want to avoid committing your database file to open source. Having it up there means that all the data you put in is also accessible to everyone on the web.
This is not a big deal for a training app, but down the road your database might contain sensible information about your users.
You can add your db.sqlite3
file to your .gitignore
file to avoid pushing it to your remote repository.
R morel on May 5, 2020
ProgrammingError at /projects/ relation “projects_project” does not exist LINE 1: …t”.”technologey”, “projects_project”.”image” FROM “projects_… ^ Request Method: GET Request URL: localhost:8000/projects/ Django Version: 2.2.1 Exception Type: ProgrammingError Exception Value: relation “projects_project” does not exist LINE 1: …t”.”technologey”, “projects_project”.”image” FROM “projects_… ^ Exception Location: /Users/morel893/Desktop/env/lib/python3.7/site-packages/django/db/backends/utils.py in _execute, line 84 Python Executable: /Users/morel893/Desktop/env/bin/python Python Version: 3.7.7 Python Path: [‘/Users/morel893/Desktop/django-portfolio’, ‘/Library/Frameworks/Python.framework/Versions/3.7/lib/python37.zip’, ‘/Library/Frameworks/Python.framework/Versions/3.7/lib/python3.7’, ‘/Library/Frameworks/Python.framework/Versions/3.7/lib/python3.7/lib-dynload’, ‘/Users/morel893/Desktop/env/lib/python3.7/site-packages’] Server time: Tue, 5 May 2020 04:33:45 +0000
help plis
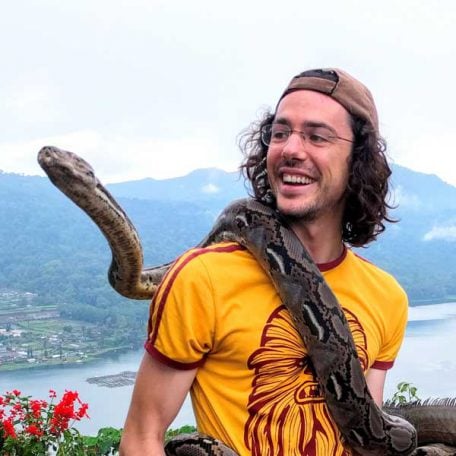
Martin Breuss RP Team on May 5, 2020
If you get a ProgrammingError
it often means that there’s something to be done with your database. Especially when it mentions “relations” like in your case.
Halp: makemigrations
and migrate
:)
Hope this helps!
R morel on May 5, 2020
ok
R morel on May 6, 2020
thanks for mi is work thanks ever
Become a Member to join the conversation.
Gascowin on Oct. 22, 2019
I found the display of projects as “Project object(n)” in the admin area to be pretty annoying and looked it up. Here is a quick workaround: In models.py modify a particular magic method to your class;
This will ensure that each project is represented by its title in the admin area.
Other nice and simple admin area customizations can be achieved by a couple one liners in admin.py: